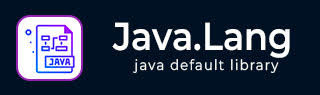
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StringBuffer append() Method
The Java StringBuffer append() method appends the string representation of its various arguments to the sequence. This method is a part of the String concatenation technique to combine two or more strings in java.
As we might already know, String concatenation technique can be done in three cases:
- Case 1 − Using the + operator
- Case 2 − Using the concat() method
- Case 3 − Using the append() method
The + operator and the append() method is the most efficient solutions for string concatenations. Also, these two cases are the same, as the JVM usually converts the case 1 to case 3 to combine strings.
Note − There are 13 different polymorphic forms of this method, all of which we will see in the following article.
Syntax
Following is the syntax for Java StringBuffer append() method
public StringBuffer append(boolean b) or, public StringBuffer append(char c) or, public StringBuffer append(char[] str) or, public StringBuffer append(char[] str, int offset, int len) or, public StringBuffer append(CharSequence s) or, public StringBuffer append(CharSequence s, int start, int end) or, public StringBuffer append(double d) or, public StringBuffer append(float f) or, public StringBuffer append(int i) or, public StringBuffer append(long lng) or, public StringBuffer append(Object obj) or, public StringBuffer append(String st) or, public StringBuffer append(StringBuffer sb)
Parameters
b − This is the boolean value.
c − This is the char value.
str − This is the characters to be appended
offset − This is the index of the first char to append.
len − This is the number of chars to append.
s − This is the CharSequence to append.
start − This is the starting index of the subsequence to be appended.
end − This is the end index of the subsequence to be appended.
d − This is the value of double.
f − This is the value of float.
i − This is the value of an int.
lng − This is the value of long.
obj − This is the value of an object.
st − This is the value of a string.
sb − This is the StringBuffer to append.
Return Value
This method returns a reference to this object.
Example
If the argument is a Boolean value, the method appends the Boolean value to the sequence directly.
The following example shows the usage of Java StringBuffer append(Boolean b) method. Here, we are instantiating a StringBuffer object "buff" with the string name "tuts". Then, we invoke the append() method using the "buff" object with a boolean argument "true". The return value will be the appended string name "tuts true". Similarly, we demonstrate another case using the string name "abcd" and a boolean argument "false".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the boolean argument as string to the string buffer buff.append(true); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the boolean argument as string to the string buffer buff.append(false); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts true buffer = abcd After append = abcd false
Example
When a char argument is passed, the method appends this argument to the contents of the input sequence. The length of this sequence increases by 1.
The following example shows the usage of Java StringBuffer append(char c) method. Here, we are creating a StringBuffer object "buff" and initializing it with the string name "tuts". Then, we invoke the append() method using the "buff" object with an argument "A". The return value will be the appended string name "tuts A". Similarly, we demonstrate another case using the string name "abcd" and a char argument "!".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the char argument as string to the string buffer. buff.append('A'); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the char argument as string to the string buffer. buff.append('!'); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts A buffer = abcd After append = abcd !
Example
When the char array is passed as an argument to the method, the characters of the array argument are appended, in order, to the contents of this sequence. The length of this sequence increases by the length of the argument.
The following example shows the usage of Java StringBuffer append(char[] str) method. Here, we are instantiating a StringBuffer object "buff" with the string name "tuts". We invoke the append() method using the "buff" object with a character array argument containing five elements {'p','o','i','n','t'}. The return value will be the appended string name "tuts point".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // char array char[] str = new char[]{'p','o','i','n','t'}; /* appends the string representation of char array argument to this string buffer */ buff.append(str); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts point
Example
When the method takes char array, an index and the length of the characters to be appended, characters of the char array starting at any index, are appended in order, to the contents of this sequence. The length of this sequence increases by the value of number of characters appended.
The following example shows the usage of Java StringBuffer append(char[] str, int offset, int len) method. We will first try to instantiate a StringBuffer object "buff" with the string name "amrood". Then, we invoke the append() method using the "buff" object with a character array argument containing 13 elements {'a','d','m','i','n','i','s','t','r','a','t','o','r'}, with an offset argument '0' and length to add as '5'. The return value will be the appended string name "amrood admin".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("amrood "); System.out.println("buffer = " + buff); // char array char[] str = new char[] {'a','d','m','i','n','i','s','t','r','a','t','o','r'}; /* appends the string representation of char array argument to this string buffer with offset at index 0 and length as 5 */ buff.append(str, 0, 5); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = amrood After append = amrood admin
Example
If a CharSequence is passed as an argument to the method, the characters of the CharSequence argument are appended in order, increasing the length of this sequence by the length of the argument.
The following example shows the usage of Java StringBuffer append(CharSequence s) method. Here, we are instantiating a StringBuffer object "buff" with the string name "compile". We invoke the append() method using the "buff" object with a CharSequence argument containing "online". The return value will be the appended string name "compile online".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("compile "); System.out.println("buffer = " + buff); CharSequence cSeq = "online"; // appends the CharSequence buff.append(cSeq); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = compile After append = compile online
Example
When a CharSequence is passed as an argument along with a start index and end index, the characters of the CharSequence argument starting at any index, are appended in order, to the contents of this sequence up to the end (exclusive) index. The length of this sequence is increased by the value of the number of characters appended.
The following example shows the usage of Java StringBuffer append(CharSequence s, int start, int end) method. We will first try to instantiate a StringBuffer object "buff" with the string name "tutorials". Then, we invoke the append() method using the "buff" object with a CharSequence argument "tutspoint", with the start argument '4' and end argument as '9'. The return value will be the appended string name "tutorials point".
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tutorials "); System.out.println("buffer = " + buff); CharSequence cSeq = "tutspoint"; // appends the CharSequence with start index 4 and end index 9 buff.append(cSeq, 4, 9); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tutorials After append = tutorials point
Example
If the argument is a double value, the method appends this double value to the sequence directly.
The following example shows the usage of Java StringBuffer append(double d) method.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the double argument as string to the string buffer buff.append(30.100000001d); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the double argument as string to the string buffer buff.append(20.12d); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts 30.100000001 buffer = abcd After append = abcd 20.12
Example
If the argument is a float value, the method appends this float value to the sequence directly.
The following example shows the usage of Java StringBuffer append(float f) method.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the float argument as string to the string buffer buff.append(6.5f); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the float argument as string to the string buffer buff.append(10.25f); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts 6.5 buffer = abcd After append = abcd 10.25
Example
If the argument is a int value, the method appends this int value to the sequence directly.
The following example shows the usage of Java StringBuffer append(int i) method.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the int argument as string to the string buffer buff.append(10); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the int argument as string to the string buffer buff.append(253); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts 10 buffer = abcd After append = abcd 253
Example
If the argument is a long value, the method appends this long value to the sequence directly.
The following example shows the usage of Java StringBuffer append(long lng) method.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tuts "); System.out.println("buffer = " + buff); // appends the long argument as string to the string buffer buff.append(254378L); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("abcd "); System.out.println("buffer = " + buff); // appends the long argument as string to the string buffer buff.append(5234l); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tuts After append = tuts 254378 buffer = abcd After append = abcd 5234
Example
If the argument is an Object value, the method appends this Object value to the sequence directly.
The following example shows the usage of Java StringBuffer append(Object obj) method. We create a StringBuffer object "buff" first and initialize it using a string name "tutorials". Then, another object is created directly using the Object class and is initialized with another string "point". These two objects are appended together by invoking the append() method using the StringBuffer object and passing another object as an argument to it.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tutorials "); System.out.println("buffer = " + buff); Object obVal = "point"; // appends the Object value buff.append(obVal); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tutorials After append = tutorials point
Example
If the argument is a String value, the method appends this String value to the sequence directly. Add four null values to the sequence if the string is null.
The following example shows the usage of Java StringBuffer append(String st) method.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tutorials "); System.out.println("buffer = " + buff); // appends the string argument to the string buffer buff.append("point"); // print the string buffer after appending System.out.println("After append = " + buff); buff = new StringBuffer("1234 "); System.out.println("buffer = " + buff); // appends the string argument to the string buffer buff.append("!#$%"); // print the string buffer after appending System.out.println("After append = " + buff); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer = tutorials After append = tutorials point buffer = 1234 After append = 1234 !#$%
Example
If the argument is a StringBuffer value, the method appends this StringBuffer value to the sequence directly. Add four null values to the sequence if the StringBuffer is null.
The following example shows the usage of Java StringBuffer append(StringBuffer sb) method. We create two StringBuffer objects "buff1" and "buff2" first. Then, initialize them using string names "tutorials" and "point" respectively. These two objects are appended together by invoking the append() method using the StringBuffer object and passing another StringBuffer object as an argument to it.
package com.tutorialspoint; import java.lang.*; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff1 = new StringBuffer("tutorials "); System.out.println("buffer1 = " + buff1); StringBuffer buff2 = new StringBuffer("point "); System.out.println("buffer2 = " + buff2); // appends stringbuffer2 to stringbuffer1 buff1.append(buff2); // print the string buffer after appending System.out.println("After append = " + buff1); } }
Output
Let us compile and run the above program, this will produce the following result −
buffer1 = tutorials buffer2 = point After append = tutorials point
Example
If both the start and end arguments of the method are negative; if start is greater than end; if end is greater than s.length(), the method throws an IndexOutOfBounds Exception in all three cases.
The following example demonstrates a scenario where the java.lang.StringBuffer.append() method throws an Exception. Here, we are instantianting a StringBuffer object "buff", and initialize a CharSequence with "tutorialspoint". Then, the method is invoked using the StringBuffer object by passing a CharSequence and the start and end arguments as negative values. The method in this case will throw an exception.
import java.lang.*; public class StringBufferAppend { public static void main(String[] args) { StringBuffer buff = new StringBuffer("tutorials "); System.out.println("buffer = " + buff); CharSequence cSeq = "tutorialspoint"; // throws exception as the index are negative buff.append(cSeq,-3, -5); System.out.println("After append = " + buff); } }
Exception
Once the program is compiled and run, the method throws an IndexOutOfBoundsException as the arguments start and end are given negative.
Exception in thread "main" java.lang.IndexOutOfBoundsException: Range [-3, -5) out of bounds for length 9 at java.base/jdk.internal.util.Preconditions$3.apply(Preconditions.java:71) at java.base/jdk.internal.util.Preconditions$3.apply(Preconditions.java:68) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.append(AbstractStringBuilder.java:683) at java.base/java.lang.StringBuffer.append(StringBuffer.java:393) at StringBufferAppend.main(StringBufferAppend.java:13)