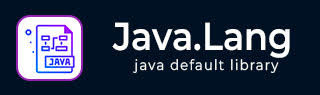
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - String substring() Method
The Java String substring() method is used to retrieve a substring of a String object. The substring starts with the character at the given index and continues to the end of the current string.
Since String is immutable in Java, this method always returns a new string, leaving the previous string untouched.
There are two polymorphic variants of this method, syntaxes of which are given below; one allows you to specify only the start index, and the other allows you to specify both the start and end indexes.
Syntax
Following is the syntax for Java String substring() method:
public String substring(int beginIndex) // first syntax or, public String substring(int beginIndex, int endIndex) // second syntax
Note − The method will return all the characters from startIndex if endIndex is not specified.
Parameters
beginIndex − This is the value of beginning index, inclusive. // first syntax
endIndex − This is the value of ending index, exclusive. // second syntax
Return Value
This method returns the specified substring.
Example
The following example shows the usage of Java String substring() method by finding the substring of the string "This is tutorials point". Here, only the begin index is passed as a argument to this method −
import java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "This is tutorials point"; String substr = ""; // prints the substring after index 7 substr = str.substring(7); System.out.println("substring = " + substr); // prints the substring after index 0 i.e whole string gets printed substr = str.substring(0); System.out.println("substring = " + substr); } }
Output
If you compile and run the above program, it will produce the following result −
substring = tutorials point substring = This is tutorials point
Example
Following is an example to show the use of substring() method in Java by finding the substring of the provided string. Here both the beginning and the ending of the string is passed as parameters −
import java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "This is tutorials point"; String substr = ""; // prints the substring after index 7 till index 17 substr = str.substring(7, 17); System.out.println("substring = " + substr); // prints the substring after index 0 till index 7 substr = str.substring(0, 7); System.out.println("substring = " + substr); } }
Output
Let us compile and run the program above, the output will be displayed as follows −
substring = tutorials substring = This is
Example
In this example we are trying to retrieve the substring with in the brackets from a string. Here, we are using indexOf() method to find the indices of both open and closed braces. Once we obtain these we are using the substring() method to get the substring within this range.
public class StringDemo { public static void main(String[] args) { String s = "Stabbed in the (back)"; // getting substring between '(' and ')' delimeter int begin = s.indexOf("("); int ter = s.indexOf(")"); String res = s.substring(begin + 1, ter); System.out.println("The substring is: " + res); } }
Output
On executing the program above, the output is obtained as follows −
The substring is: back
Example
Till now we have used index values to determine the boundaries of a desired substring. We can also print a substring existing between two given string values. Following is an example to do so −
public class StringDemo { public static void main(String[] args) { String s = "Stabbed in the (back)"; // getting substring between '(' and ')' delimeter int begin = s.indexOf("(") + 1; int ter = s.indexOf(")"); String res = s.substring(begin, ter); System.out.println("The substring is: " + res); } }
Output
Following is the output of the above program −
The substring is: back
Example
The substring() method is used in this program to remove the first and last characters from a string.
public class StringDemo { public static void main(String args[]) { String s = new String("Programming"); System.out.print("Substring after eliminating the first character is: "); // excluding the first character since index(0) is excluded System.out.println(s.substring(1)); System.out.print("Substring after eliminating the last character is: "); // excluding the last character by providing index as -1 System.out.println(s.substring(0, s.length() - 1)); System.out.println( "Substring after eliminating both the first and the last character is: " + s.substring(1, s.length() - 1)); } }
Output
Following is an output of the above code −
Substring after eliminating the first character is: rogramming Substring after eliminating the last character is: Programmin Substring after eliminating both the first and the last character is: rogrammin