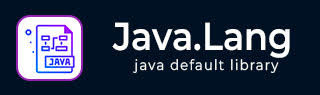
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Float compareTo() method
Description
The Java Float compareTo() method compares two Float objects numerically. There are two ways in which comparisons performed by this method differ from those performed by the Java language numerical comparison operators (<, <=, ==, >= >) when applied to primitive float values −
- Float.NaN is considered by this method to be equal to itself and greater than all other float values (including Float.POSITIVE_INFINITY).
- 0.0f is considered by this method to be greater than -0.0f.
Declaration
Following is the declaration for java.lang.Float.compareTo() method
public int compareTo(Float anotherFloat)
Parameters
anotherFloat − This is the Float to be compared.
Return Value
This method returns the value 0 if anotherFloat is numerically equal to this Float; a value less than 0 if this Float is numerically less than anotherFloat; and a value greater than 0 if this Float is numerically greater than anotherFloat.
Exception
NA
Example 1
The following example shows the usage of Float compareTo() method to check a value is greater than another value or not. We're having two Float objects and using compareTo() method, we're comparing these float objects and then result is compared to 0. If result is greater than 0 then first number is greater than second number. If result is less than 0 then first number is less than second number. Otherwise both values are same.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // compareTos the two specified float objects Float f1 = new Float("11.50"); Float f2 = new Float("8.50"); int retval = f1.compareTo(f2); if(retval > 0) { System.out.println("f1 is greater than f2"); } else if(retval < 0) { System.out.println("f1 is less than f2"); } else { System.out.println("f1 is equal to f2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
f1 is greater than f2
Example 2
The following example shows the usage of Float compareTo() method to check a value is less than another value or not. We're having two Float objects and using compareTo() method, we're comparing these float objects and then result is compared to 0. If result is greater than 0 then first number is greater than second number. If result is less than 0 then first number is less than second number. Otherwise both values are same.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // compareTos the two specified float values Float f1 = new Float("8.50"); Float f2 = new Float("11.50"); int retval = f1.compareTo(f2); if(retval > 0) { System.out.println("f1 is greater than f2"); } else if(retval < 0) { System.out.println("f1 is less than f2"); } else { System.out.println("f1 is equal to f2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
f1 is less than f2
Example 3
The following example shows the usage of Float compareTo() method to check a value is same as another value or not. We're having two Float objects and using compareTo() method, we're comparing these float objects and then result is compared to 0. If result is greater than 0 then first number is greater than second number. If result is less than 0 then first number is less than second number. Otherwise both values are same.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // compareTos the two specified float values Float f1 = new Float("11.50"); Float f2 = new Float("11.50"); int retval = f1.compareTo(f2); if(retval > 0) { System.out.println("f1 is greater than f2"); } else if(retval < 0) { System.out.println("f1 is less than f2"); } else { System.out.println("f1 is equal to f2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
f1 is equal to f2