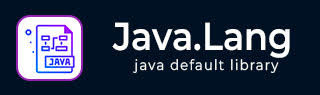
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Enum hashCode() method
Description
The Java Enum hashCode() method returns a hash code for this enum constant.
Declaration
Following is the declaration for java.lang.Enum.hashCode() method
public final int hashCode()
Parameters
NA
Return Value
This method returns a hash code for this enum constant.
Exception
NA
Example 1
The following example shows the usage of hashCode() method for an enum.
package com.tutorialspoint; // enum showing programming languages enum Language { C, Java, PHP; } public class EnumDemo { public static void main(String args[]) { // returns the name and hashCode of this enum constant System.out.print("Programming in " + Language.C.toString()); System.out.println(", Hashcode = " + Language.C.hashCode()); System.out.print("Programming in " + Language.Java.toString()); System.out.println(", Hashcode = " + Language.Java.hashCode()); System.out.print("Programming in " + Language.PHP.toString()); System.out.println(", Hashcode = " + Language.PHP.hashCode()); } }
Output
Let us compile and run the above program, this will produce the following result −
Programming in C, Hashcode = 112810359 Programming in Java, Hashcode = 205029188 Programming in PHP, Hashcode = 1309552426
Example 2
The following example shows the another usage of hashCode() method for a different enum.
package com.tutorialspoint; // enum showing topics covered under Tutorials enum Tutorials { Java, HTML, Python; } public class EnumDemo { public static void main(String args[]) { Tutorials t1, t2, t3; t1 = Tutorials.Java; t2 = Tutorials.HTML; t3 = Tutorials.Python; System.out.print("Programming in " + t1.toString()); System.out.println(", Hashcode = " + t1.hashCode()); System.out.print("Programming in " + t2.toString()); System.out.println(", Hashcode = " + t2.hashCode()); System.out.print("Programming in " + t3.toString()); System.out.println(", Hashcode = " + t3.hashCode()); } }
Output
Let us compile and run the above program, this will produce the following result −
Programming in Java, Hashcode = 112810359 Programming in HTML, Hashcode = 205029188 Programming in Python, Hashcode = 1309552426
java_lang_enum.htm
Advertisements
To Continue Learning Please Login