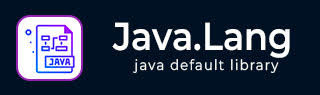
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Character isSurrogatePair() Method
Description
The Java Character isSurrogatePair() method determines whether the pair of char values is a valid Unicode surrogate pair or not.
Let us first understand what surrogate pairs are and how they are useful in various scenarios −
A “surrogate” is a UTF – 16 value that is used to represent a singular supplementary character. A supplementary character is a Unicode value that does not have a valid representation in the Unicode system; hence, a pair of such surrogate characters, called the surrogate pairs, are used to represent these values.
The first value in the pair is known as the high (or leading) surrogate value and its next value is known as the low (or trailing) surrogate value. 16-bit High surrogate code point value falls in the range U+D800 to U+DBFF, whereas the 16-bit low surrogate code point value falls in the range U+DC00 to U+DFFF.
Syntax
Following is the syntax for Java Character isSurrogatePair() method
public static boolean isSurrogatePair(char high, char low)
Parameters
high − the high-surrogate code value to be tested
low − the low-surrogate code value to be tested
Return Value
This method returns true if the specified high and low-surrogate code values represent a valid surrogate pair, false otherwise.
Checking a char pair to be Surrogate pair of code unit Example
The following example shows the usage of Java Character isSurrogatePair() method. In this example, we've created two char variables and assigned it two unicode values. Then using isSurrogatePair() method, we've checked the char pair and result is printed.
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create 2 char primitives ch1, ch2 char ch1, ch2; // assign values to ch1, ch2 ch1 = '\ud800'; ch2 = '\udc00'; // create a boolean primitive b boolean b; // check if ch1, ch2 is a surrogate pair and assign result to b b = Character.isSurrogatePair(ch1, ch2); String str = "ch1, ch2 is a valid Unicode surrogate pair is " + b; // print b value System.out.println( str ); } }
Output
Let us compile and run the above program, this will produce the following result −
ch1, ch2 is a valid Unicode surrogate pair is true
Checking a char pair to be Surrogate pair of code unit Example
The following example shows the usage of Java Character isSurrogatePair() method. In this example, we've created two char variables and assigned it two unicode values. Then using isSurrogatePair() method, we've checked the char pair and result is printed.
package com.tutorialspoint; public class SurrogatePairDemo { public static void main(String[] args) { char c1 = '\ud800'; char c2 = '\u4563'; boolean b = Character.isSurrogatePair(c1, c2); System.out.println(c1 + " and " + c2 + " are a surrogate pair is " + b); } }
Output
The output after compiling and executing the program will be displayed as follows −
? and ? are a surrogate pair is false
Checking a char pair to be Surrogate pair of code unit Example
We can also use conditional statements to pass the Boolean return value of the method as the condition to it. Let us see an example that does the same.
package com.tutorialspoint; public class SurrogatePairDemo { public static void main(String[] args) { char c1 = '\ud801'; char c2 = '\udc3f'; boolean b = Character.isSurrogatePair(c1, c2); if(b) System.out.println("The characters are a surrogate pair"); else System.out.println("The characters are not a surrogate pair"); } }
Output
The output of the given program will be displayed as follows −
The characters are a surrogate pair
Checking a char pair to be Surrogate pair of code unit Example
In another example, we declare and initialize two character arrays and use loop statements to check whether the corresponding elements in these two arrays are surrogate pairs or not.
package com.tutorialspoint; public class SurrogatePairDemo { public static void main(String []args){ char cp1[] = new char[] {'\ud810', '\ud967', '\ud832', '\u7843'}; char cp2[] = new char[] {'\u5637', '\udc10', '\udfff', '\udd56'}; for(int i = 0; i < cp1.length; i++) { if(Character.isSurrogatePair(cp1[i], cp2[i])) System.out.println("The character is a surrogate pair"); else System.out.println("The character is not a surrogate pair"); } } }
Output
Let us compile and run the program given above before the output is printed as follows −
The character is not a surrogate pair The character is a surrogate pair The character is a surrogate pair The character is not a surrogate pair
To Continue Learning Please Login