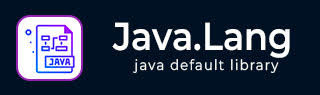
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java.lang.Character.getNumericValue() Method
Description
The java.lang.Character.getNumericValue(int codePoint) returns the int value that the specified character (Unicode code point) represents. For example, the character '\u216C' (the Roman numeral fifty) will return an int with a value of 50.
The letters A-Z in their uppercase ('\u0041' through '\u005A'), lowercase ('\u0061' through '\u007A'), and full width variant ('\uFF21' through '\uFF3A' and '\uFF41' through '\uFF5A') forms have numeric values from 10 through 35. This is independent of the Unicode specification, which does not assign numeric values to these char values.
If the character does not have a numeric value, then -1 is returned. If the character has a numeric value that cannot be represented as a nonnegative integer (for example, a fractional value), then -2 is returned.
Declaration
Following is the declaration for java.lang.Character.getNumericValue() method
public static int getNumericValue(int codePoint)
Parameters
codePoint − the character (Unicode code point) to be converted
Return Value
This method returns the numeric value of the character, as a nonnegative int value; -2 if the character has a numeric value that is not a nonnegative integer; -1 if the character has no numeric value.
Exception
NA
Example
The following example shows the usage of lang.Character.getNumericValue() method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 2 int primitives cp1, cp2 int cp1, cp2; // assign values to cp1, cp2 cp1 = 0x0068; cp2 = 0xff30; // create 2 int primitives i1, i2 int i1, i2; // assign numeric values of cp1, cp2 to i1, i2 i1 = Character.getNumericValue(cp1); i2 = Character.getNumericValue(cp2); String str1 = "Numeric value of code point cp1 is " + i1; String str2 = "Numeric value of code point cp2 is " + i2; // print i1, i2 values System.out.println( str1 ); System.out.println( str2 ); } }
Let us compile and run the above program, this will produce the following result −
Numeric value of code point cp1 is 17 Numeric value of code point cp2 is 25