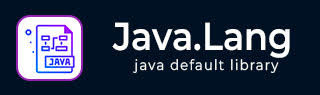
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Character getNumericValue Method
The Java Character getNumericValue() method is used to get the integer value that a specified Unicode character represents. For example, the character '\u216C' (the roman numeral fifty) will return an integer with a value of 50.
The letters A-Z in their uppercase ('\u0041' through '\u005A'), lowercase ('\u0061' through '\u007A'), and full width variant ('\uFF21' through '\uFF3A' and '\uFF41' through '\uFF5A') forms have numeric values from 10 through 35. This is independent of the Unicode specification, which does not assign numeric values to these char values.
Note − Extreme Characters (Character.MAX_VALUE and Character.MIN_VALUE) hold no numeric values.
Syntax
Following is the syntax for Java Character getNumericValue() method
public static int getNumericValue(char ch) or, public static int getNumericValue(int codePoint)
Parameters
ch − the character to be converted
codePoint − the Unicode code point to be converted
Return Value
This method returns the numeric value of the character, as a non-negative int value; -2 if the character has a numeric value that is not a non-negative integer; -1 if the character has no numeric value.
Example
The following example shows the usage of Java Character getNumericValue(char ch) method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 2 character primitives ch1, ch2 char ch1, ch2; // assign values to ch1, ch2 ch1 = 'j'; ch2 = '4'; // create 2 int primitives i1, i2 int i1, i2; // assign numeric values of ch1, ch2 to i1, i2 i1 = Character.getNumericValue(ch1); i2 = Character.getNumericValue(ch2); String str1 = "Numeric value of " + ch1 + " is " + i1; String str2 = "Numeric value of " + ch2 + " is " + i2; // print i1, i2 values System.out.println( str1 ); System.out.println( str2 ); } }
Output
Let us compile and run the above program, this will produce the following result −
Numeric value of j is 19 Numeric value of 4 is 4
Example
The following example shows the usage of Java Character getNumericValue(int codePoint) method.
import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 2 int primitives cp1, cp2 int cp1, cp2; // assign values to cp1, cp2 cp1 = 0x0068; cp2 = 0xff30; // create 2 int primitives i1, i2 int i1, i2; // assign numeric values of cp1, cp2 to i1, i2 i1 = Character.getNumericValue(cp1); i2 = Character.getNumericValue(cp2); // print i1, i2 values System.out.println("Numeric value of code point cp1 is " + i1); System.out.println("Numeric value of code point cp2 is " + i2); } }
Output
Let us compile and run the above program, this will produce the following result −
Numeric value of code point cp1 is 17 Numeric value of code point cp2 is 25
Example
In another example, we pass integers that are not in a code point format as arguments to this method.
import java.lang.*; public class CharacterDemo { public static void main(String[] args) { int cp1, cp2, cp3; cp1 = 74893; cp2 = 100; cp3 = 0; System.out.println("Numeric value of code point cp1 is " + Character.getNumericValue(cp1)); System.out.println("Numeric value of code point cp2 is " + Character.getNumericValue(cp2)); System.out.println("Numeric value of code point cp3 is " + Character.getNumericValue(cp3)); } }
Output
If we compile and run the program, the output is obtained as −
Numeric value of code point cp1 is -1 Numeric value of code point cp2 is 13 Numeric value of code point cp3 is -1
Example
Symbols are non-numeric values. Hence, when they are passed as arguments to the method, -1 is returned. Let us see the program below −
import java.lang.*; public class CharacterDemo { public static void main(String args[]) { char c1 = '%'; char c2 = '&'; char c3 = '#'; int res = Character.getNumericValue(c1); System.out.println("The numeric value of given character is " + res); res = Character.getNumericValue(c2); System.out.println("The numeric value of given character is " + res); res = Character.getNumericValue(c3); System.out.println("The numeric value of given character is " + res); } }
Output
The program above is to be compiled and run to obtain the output given as follows −
The numeric value of given character is -1 The numeric value of given character is -1 The numeric value of given character is -1