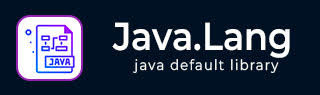
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Character codePointAt() Method
The Java Character codePointAt() will retrieve the code point of a character present at an index of a char array. These code points can represent ordinary character and supplementary characters as well.
Supplementary code point characters will be obtained when the value at a certain index in the char array satisfies the given conditions below −
If the char value belongs to the high-surrogate range (U+D800 to U+DBFF).
If the following index of the given index is less than the length of the char array.
If the value in this following index belongs to the low surrogate range (U+DC00 to U+DFFF).
Ordinary code point characters will be obtained in any other case.
Note − This method occurs in three different polymorphic forms with different parameters.
Syntax
Following are the various syntaxes for Java Character codePointAt() method
public static int codePointAt(char[] a, int index) (or) public static int codePointAt(char[] a, int index, int limit) (or) public static int codePointAt(CharSequence seq, int index)
Parameters
a − The char array
index − The index to the char values (Unicode code units) in the char array to be converted
limit − The index after the last array element that can be used in the char array.
seq − A sequence of char values (Unicode code units)
Return Value
This method returns the Unicode code point at the given index of the char array/CharSequence argument.
Example
When we take a simple character array and a specified index less than the array length as the arguments for the method, the return value will be the code point of the character present in that index.
The following example shows the usage of the Java Character codePointAt() method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create 2 int's res, index and assign value to index int res, index = 0; // assign result of codePointAt on array c at index to res res = Character.codePointAt(c, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
Let us compile and run the above program, this will produce the following result −
Unicode code point is 97
Example
In another example below, we use loop statements to retrieve the code point of each element in an array. The code point of every element in the given character array is retrieved by passing every index as the argument to the method using loop statements.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c_arr = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create 2 int's res, index and assign value to index int res, index; // using a for loop assign the codePointAt on array c at index to res for (index = 0; index < c_arr.length; index++){ res = Character.codePointAt(c_arr, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } } }
If you compile the code above, the output is obtained as:
Unicode code point is 97 Unicode code point is 98 Unicode code point is 99 Unicode code point is 100 Unicode code point is 101
Example
We can restrict the search in this char array by passing an additional parameter 'limit'. In this scenario, the method will only perform the search upto the given limit and returns the code point.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create and assign value to integers index, limit int index = 1, limit = 3; // create an int res int res; // assign result of codePointAt on array c at index to res using limit res = Character.codePointAt(c, index, limit); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
Let us compile and run the above program, this will produce the following result −
Unicode code point is 98
Example
However, if the index argument is greater than the length of the array argument or the limit argument, the method throws an IndexOutOfBounds Exception.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] {'a', 'b', 'c', 'd', 'e'}; // assign an index value greater than array length int res, index = 6; // assign result of codePointAt on array c at index to res res = Character.codePointAt(c, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
Exception
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 6at java.lang.Character.codePointAtImpl(Character.java:4952) at java.lang.Character.codePointAt(Character.java:4915) at com.tutorialspoint.CharacterDemo.main(CharacterDemo.java:16)
Example
When we pass a CharSequence and an index value as the arguments to the codePointAt(CharSequence seq, int index) method, the return value is the character present at the index in the given CharSequence.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a CharSequence seq and assign value CharSequence seq = "Hello"; // create and assign value to index int index = 4; // create an int res int res; // assign result of codePointAt on seq at index to res res = Character.codePointAt(seq, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
Let us compile and run the above program, this will produce the following result −
Unicode code point is 111
Example
But if the CharSequence is initialized with a null value and passed as an argument to the method, a NullPointer Exception is raised.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create a CharSequence seq and assign value CharSequence seq = null; // create and assign value to index int index = 5; // create an int res int res; // assign result of codePointAt on seq at index to res res = Character.codePointAt(seq, index); // print res value System.out.println("Unicode code point is " + res); } }
Exception
Exception in thread "main" java.lang.NullPointerExceptionat java.lang.Character.codePointAt(Character.java:4883)
To Continue Learning Please Login