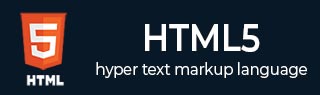
- HTML5 Tutorial
- HTML5 - Home
- HTML5 - Overview
- HTML5 - Syntax
- HTML5 - Attributes
- HTML5 - Events
- HTML5 - Web Forms 2.0
- HTML5 - SVG
- HTML5 - MathML
- HTML5 - Web Storage
- HTML5 - Web SQL Database
- HTML5 - Server-Sent Events
- HTML5 - WebSocket
- HTML5 - Canvas
- HTML5 - Audio & Video
- HTML5 - Geolocation
- HTML5 - Microdata
- HTML5 - Drag & drop
- HTML5 - Web Workers
- HTML5 - IndexedDB
- HTML5 - Web Messaging
- HTML5 - Web CORS
- HTML5 - Web RTC
- HTML5 Demo
- HTML5 - Web Storage
- HTML5 - Server Sent Events
- HTML5 - Canvas
- HTML5 - Audio Players
- HTML5 - Video Players
- HTML5 - Geo-Location
- HTML5 - Drag and Drop
- HTML5 - Web Worker
- HTML5 - Web slide Desk
- HTML5 Tools
- HTML5 - SVG Generator
- HTML5 - MathML
- HTML5 - Velocity Draw
- HTML5 - QR Code
- HTML5 - Validator.nu Validation
- HTML5 - Modernizr
- HTML5 - Validation
- HTML5 - Online Editor
- HTML5 - Color Code Builder
- HTML5 Useful References
- HTML5 - Quick Guide
- HTML5 - Color Names
- HTML5 - Fonts Reference
- HTML5 - URL Encoding
- HTML5 - Entities
- HTML5 - Char Encodings
- HTML5 Tag Reference
- HTML5 - Question and Answers
- HTML5 - Tags Reference
- HTML5 - Deprecated Tags
- HTML5 - New Tags
- HTML5 Resources
- HTML5 - Useful Resources
- HTML5 - Discussion
HTML5 Canvas - Drawing Lines
Line Methods
We require the following methods to draw lines on the canvas −
Sr.No. | Method and Description |
---|---|
1 | beginPath() This method resets the current path. |
2 | moveTo(x, y) This method creates a new subpath with the given point. |
3 | closePath() This method marks the current subpath as closed, and starts a new subpath with a point the same as the start and end of the newly closed subpath. |
4 | fill() This method fills the subpaths with the current fill style. |
5 | stroke() This method strokes the subpaths with the current stroke style. |
6 | lineTo(x, y) This method adds the given point to the current subpath, connected to the previous one by a straight line. |
Example
Following is a simple example which makes use of the above-mentioned methods to draw a triangle.
<!DOCTYPE HTML> <html> <head> <style> #test { width: 100px; height:100px; margin: 0px auto; } </style> <script type = "text/javascript"> function drawShape() { // get the canvas element using the DOM var canvas = document.getElementById('mycanvas'); // Make sure we don't execute when canvas isn't supported if (canvas.getContext) { // use getContext to use the canvas for drawing var ctx = canvas.getContext('2d'); // Filled triangle ctx.beginPath(); ctx.moveTo(25,25); ctx.lineTo(105,25); ctx.lineTo(25,105); ctx.fill(); // Stroked triangle ctx.beginPath(); ctx.moveTo(125,125); ctx.lineTo(125,45); ctx.lineTo(45,125); ctx.closePath(); ctx.stroke(); } else { alert('You need Safari or Firefox 1.5+ to see this demo.'); } } </script> </head> <body id = "test" onload = "drawShape();"> <canvas id = "mycanvas"></canvas> </body> </html>
The above example would draw following shape −
Line Properties
There are several properties which allow us to style lines.
S.No. | Property and Description |
---|---|
1 | lineWidth [ = value ] This property returns the current line width and can be set, to change the line width. |
2 | lineCap [ = value ] This property returns the current line cap style and can be set, to change the line cap style. The possible line cap styles are butt, round, and square |
3 | lineJoin [ = value ] This property returns the current line join style and can be set, to change the line join style. The possible line join styles are bevel, round, andmiter. |
4 | miterLimit [ = value ] This property returns the current miter limit ratio and can be set, to change the miter limit ratio. |
Example
Following is a simple example which makes use of lineWidth property to draw lines of different width.
<!DOCTYPE HTML> <html> <head> <style> #test { width: 100px; height:100px; margin: 0px auto; } </style> <script type = "text/javascript"> function drawShape() { // get the canvas element using the DOM var canvas = document.getElementById('mycanvas'); // Make sure we don't execute when canvas isn't supported if (canvas.getContext) { // use getContext to use the canvas for drawing var ctx = canvas.getContext('2d'); for (i=0;i<10;i++){ ctx.lineWidth = 1+i; ctx.beginPath(); ctx.moveTo(5+i*14,5); ctx.lineTo(5+i*14,140); ctx.stroke(); } } else { alert('You need Safari or Firefox 1.5+ to see this demo.'); } } </script> </head> <body id = "test" onload = "drawShape();"> <canvas id = "mycanvas"></canvas> </body> </html>
The above example would draw following shape −