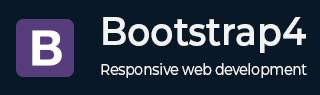
- Bootstrap 4 Tutorial
- Bootstrap 4 - Home
- Bootstrap 4 - Overview
- Bootstrap 4 - Environment Setup
- Bootstrap 4 - Layout
- Bootstrap 4 - Grid System
- Bootstrap 4 - Content
- Bootstrap 4 - Components
- Bootstrap 4 - Utilities
- Differences Between Bootstrap 3 and 4
- Bootstrap 4 Useful Resources
- Bootstrap 4 - Quick Guide
- Bootstrap 4 - Useful Resources
- Bootstrap 4 - Discussion
Bootstrap 4 - Pagination
Description
Pagination is used to divide the related content across multiple pages. The basic pagination can be created by using .pagination class to an <ul> element.
The following example demonstrates this −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <h2>Basic Pagination</h2> <nav> <ul class = "pagination"> <li class = "page-item"> <a class = "page-link" href = "#">Previous</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">4</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">5</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
Active and Disabled States
You can specify the current page by adding .active class and disable the option by adding .disabled class to the <li> element. The following example demonstrates use of .active and .disabled classes −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <h2>Active State</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination"> <li class = "page-item"> <a class = "page-link" href = "#">Previous</a> </li> <li class = "page-item "> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item active"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> <br> <h2>Disabled State</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination"> <li class = "page-item"> <a class = "page-link" href = "#">Previous</a> </li> <li class = "page-item "> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item disabled"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
Pagination Sizing
Use the .pagination-lg class to create large pagination and .pagination-sm class to create small pagination.
The following example demonstrates this −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <h2>Large Pagination</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination pagination-lg"> <li class = "page-item"> <a class = "page-link" href = "#">Previous</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> <br> <h2>Small Pagination</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination pagination-sm"> <li class = "page-item"> <a class = "page-link" href = "#">Previous</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
Pagination Alignment
Pagination can be aligned to the center by adding the .justify-content-center class and to the right side by adding .justify-content-end class as shown in the below example −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <h2>Center Aligned Pagination</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination justify-content-center"> <li class = "page-item"> <a class = "page-link" href = "#" tabindex = "-1">Previous</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> <br> <h2>Right Aligned Pagination</h2> <nav aria-label = "Page navigation example"> <ul class = "pagination justify-content-end"> <li class = "page-item"> <a class = "page-link" href = "#" tabindex = "-1">Previous</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">1</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">2</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">3</a> </li> <li class = "page-item"> <a class = "page-link" href = "#">Next</a> </li> </ul> </nav> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −