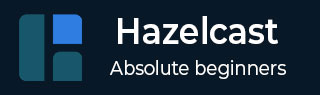
- Hazelcast Tutorial
- Hazelcast - Home
- Hazelcast - Introduction
- Hazelcast - Setup
- Hazelcast - First Application
- Hazelcast - Configuration
- Setting up multi-node instances
- Hazelcast - Data Structures
- Hazelcast - Client
- Hazelcast - Serialization
- Hazelcast Advanced
- Hazelcast - Spring Integration
- Hazelcast - Monitoring
- Map Reduce & Aggregations
- Hazelcast - Collection Listener
- Common Pitfalls & Performance Tips
- Hazelcast Useful Resources
- Hazelcast - Quick Guide
- Hazelcast - Useful Resources
- Hazelcast - Discussion
Hazelcast - ISet
The java.util.Set provides an interface for holding collections of objects which are unique. The ordering of elements does not matter.
Similarly, ISet implements a distributed version of Java Set. It provides similar functions: add, forEach, etc.
One important point to note about ISet is that, unlike other collection data, it is not partitioned. All the data is stored/present on a single JVM. Data is still accessible to all JVMs, but the set cannot be scaled beyond a single machine/JVM.
The set supports synchronous backup as well as asynchronous backup. Synchronous backup ensures that even if the JVM holding the set goes down, all elements would be preserved and available from the backup.
Let's look at an example of the useful functions.
Adding elements and reading elements
Let’s execute the following code on 2 JVMs. The producer code on one and consumer code on other.
Example
The first piece is the producer code which creates a set and adds item to it.
public static void main(String... args) throws IOException, InterruptedException { //initialize hazelcast instance HazelcastInstance hazelcast = Hazelcast.newHazelcastInstance(); // create a set ISet<String> hzFruits = hazelcast.getSet("fruits"); hzFruits.add("Mango"); hzFruits.add("Apple"); hzFruits.add("Banana"); // adding an existing fruit System.out.println(hzFruits.add("Apple")); System.out.println("Size of set:" + hzFruits.size()); System.exit(0); }
The second piece is of consumer code which reads set elements.
public static void main(String... args) throws IOException, InterruptedException { //initialize hazelcast instance HazelcastInstance hazelcast = Hazelcast.newHazelcastInstance(); // create a set ISet<String> hzFruits = hazelcast.getSet("fruits"); Thread.sleep(2000); hzFruits.forEach(System.out::println); System.exit(0); }
Output
The output for the code for the producer shows that it is not able to add an existing element.
false 3
The output for the code for the consumer prints set size and the fruits which are can be in a different order.
3 Banana Mango Apple
Useful Methods
Sr.No | Function Name & Description |
---|---|
1 | add(Type element) Add element to the set if not already present |
2 | remove(Type element) Remove element from the set |
3 | size() Return the count of elements in the set |
4 | contains(Type element) Return if the element is present |
5 | getPartitionKey() Return the partition key which hold the set |
6 | addItemListener(ItemListener<Type>listener, value) Notifies the subscriber of an element being removed/added/modified in the set. |