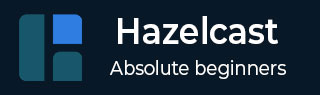
- Hazelcast Tutorial
- Hazelcast - Home
- Hazelcast - Introduction
- Hazelcast - Setup
- Hazelcast - First Application
- Hazelcast - Configuration
- Setting up multi-node instances
- Hazelcast - Data Structures
- Hazelcast - Client
- Hazelcast - Serialization
- Hazelcast Advanced
- Hazelcast - Spring Integration
- Hazelcast - Monitoring
- Map Reduce & Aggregations
- Hazelcast - Collection Listener
- Common Pitfalls & Performance Tips
- Hazelcast Useful Resources
- Hazelcast - Quick Guide
- Hazelcast - Useful Resources
- Hazelcast - Discussion
Hazelcast - ICountDownLatch
The java.util.concurrent.CountDownLatch provides a way for threads to wait, while other threads complete a set of operations in a multithreaded environment in a JVM.
Similarly, ICountDownLatch provides a distributed version of Java CountDownLatch. It provides similar functions: setCount, countDown, await, etc.
A major difference between ICountDownLatch and Java CountDownLatch is that while Java CountDownLatch provides protection of critical section from threads in a single JVM, ICountDownLatch provides synchronization for threads in a single JVM as well as multiple JVMs.
ICountDownLatch has one synchronous backup which means if we have a setup where we have, say, 5 JVMs running, only two JVMs will hold this latch.
Setting Latch & Awaiting Latch
Let’s execute the following code on two JVMs. The master code on one and worker code on other. The code is supposed to make the worker thread wait till the master thread completes.
Example
The first piece is the master code which creates a latch and counts it down.
public static void main(String... args) throws IOException, InterruptedException { //initialize hazelcast instance HazelcastInstance hazelcast=Hazelcast.newHazelcastInstance(); // create a lock ICountDownLatch countDownLatch=hazelcast.getCountDownLatch("count_down_1"); System.out.println("Setting counter"); countDownLatch.trySetCount(2); Thread.sleep(2000); System.out.println("Counting down"); countDownLatch.countDown(); Thread.sleep(2000); System.out.println("Counting down"); countDownLatch.countDown(); System.exit(0); }
The second piece is of worker code which creates a latch and counts it down.
public static void main(String... args) throws IOException, InterruptedException { //initialize hazelcast instance HazelcastInstance hazelcast = Hazelcast.newHazelcastInstance(); // create a lock ICountDownLatch countDownLatch=hazelcast.getCountDownLatch("count_down_1"); countDownLatch.await(5000, TimeUnit.MILLISECONDS); System.out.println("Worker successful"); System.exit(0); }
Output
The output for the code shows that the worker prints only after the countdown was completed to 0.
Setting counter Counting down Counting down Worker successful
Useful Methods
Sr.No | Function Name & Description |
---|---|
1 | await() Wait for the latch’s count to reach to zero before proceeding |
2 | countDown() Decrement the countdown latch |
3 | trySetCount(int count) Set the count of the latch |
4 | getCount() Get the current count of the latch |