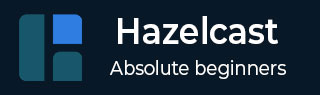
- Hazelcast Tutorial
- Hazelcast - Home
- Hazelcast - Introduction
- Hazelcast - Setup
- Hazelcast - First Application
- Hazelcast - Configuration
- Setting up multi-node instances
- Hazelcast - Data Structures
- Hazelcast - Client
- Hazelcast - Serialization
- Hazelcast Advanced
- Hazelcast - Spring Integration
- Hazelcast - Monitoring
- Map Reduce & Aggregations
- Hazelcast - Collection Listener
- Common Pitfalls & Performance Tips
- Hazelcast Useful Resources
- Hazelcast - Quick Guide
- Hazelcast - Useful Resources
- Hazelcast - Discussion
Hazelcast - IAtomicLong
The Atomic Long data structure in Java provides a thread safe way for using Long.
Similarly, IAtomicLong is more of a distributed version of AtomicLong. It provides similar functions of which following are useful ones: set, get, getAndSet, incrementAndGet. One important point to note here is that the performance of the above functions may not be similar as the data structure is distributed across machines.
AtomicLong has one synchronous backup which means if we have a setup where we have, say, 5 JVMs running, only two JVMs will hold this variable.
Let's look at some of the useful functions.
Initializing and Setting value to IAtomicLong
Example
public class Application { public static void main(String... args) throws IOException { //initialize hazelcast instance and the counter variable Hazelcast – Data Structures HazelcastInstance hazelcast = Hazelcast.newHazelcastInstance(); IAtomicLong counter = hazelcast.getAtomicLong("counter"); System.out.println(counter.get()); counter.set(2); System.out.println(counter.get()); System.exit(0); } }
Output
When the above code is executed, it will produce the following output
0 2
Synchronization across JVMs
Atomic Long provides concurrency control across JVMs. So, methods like incrementAndGet, compareAndSet can be used to atomically update the counter
Example
Let’s execute code below simultaneously, on two JVMs
public class AtomicLong2 { public static void main(String... args) throws IOException, InterruptedException { // initialize hazelcast instance HazelcastInstance hazelcast = Hazelcast.newHazelcastInstance(); IAtomicLong counter = hazelcast.getAtomicLong("counter"); for(int i = 0; i < 1000; i++) { counter.incrementAndGet(); } System.exit(0); } }
Output
The 2nd line of the output of the above code would always be −
2000
If incrementAndGet() would not have been thread safe, the above code may have not given 2000 as the output all the time. It would probably be less than that, as the writes one thread may have gotten overwritten by another.
Useful Methods
Sr.No | Function Name & Description |
---|---|
1 | get() Return the current value |
2 | set(long newValue) Set the value to newValue |
3 | addAndGet(long value) Atomically add the value and return the updated value |
4 | decrementAndGet(long value) Atomically subtract the value and return the updated value |
5 | getAndAdd(long value) Atomically return the current value and store the sum of current value and the value |
6 | getAndDecrement(long value) Atomically return the current value and store the subtraction of value from the current value |
7 | compareAndSet(long expected,long newValue) Atomically set value to newValue if the oldValue is equal to expected value |
8 | decrementAndGet(long value) Atomically subtract the value and return the updated value |