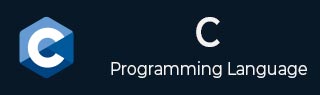
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - strchr() function
The C Library strchr() function refer to first occurence of a character from a given string. This function operate the task of null characters(\0) or null-ended string. This function is useful in a variety of text processing tasks where user need to locate a particular character.
Syntax
Following is the syntax of the C library strchr() function −
char *strchr(const char *str, int search_str)
Parameters
This function accepts the following parameters−
str − This parameter represent a given string.
search_str − This parameter refers to specific character to be searched in a given string.
Return Value
This function returns a pointer to the first occurrence of the character i.e. "search_str" in the string(str), or NULL if the character is not found.
Example 1
Following is the basic C library program that illustrate the character search in a given string using the strchr() function.
#include <stdio.h> #include <string.h> int main () { const char str[] = "Tutorialspoint"; // "ch" is search string const char ch = '.'; char *ret; ret = strchr(str, ch); printf("String after |%c| is - |%s|\n", ch, ret); return(0); }
Output
On execution of above code, we get the result value as null because the character '.' was not found in the string.
String after |.| is - |(null)|
Example 2
Here, we have a specific character to determine the extraction of substring from a given string.
#include <stdio.h> #include <string.h> int main() { const char *str = "Welcome to Tutorialspoint"; char ch = 'u'; // Find the first occurrence of 'u' in the string char *p = strchr(str, ch); if (p != NULL) { printf("String starting from '%c' is: %s\n", ch, p); } else { printf("Character '%c' not found in the string.\n", ch); } return 0; }
Output
The above code produces the following result−
String starting from 'u' is: utorialspoint
Example 3
In this example, we will find the position of character from a given string.
#include <stdio.h> #include <string.h> int main() { char str[] = "This is simple string"; char* sh; printf("Searching for the character in 's' in the given string i.e. \"%s\"\n", str); sh = strchr(str, 's'); while (sh != NULL) { printf("Found at position- %d\n", sh - str + 1); sh = strchr(sh + 1, 's'); } return 0; }
Output
On execution of above code, we observed that the character 'S' appears 4th times in different positions of the given string.
Searching for the character in 's' in the given string i.e. "This is simple string" Found at position- 4 Found at position- 7 Found at position- 9 Found at position- 16