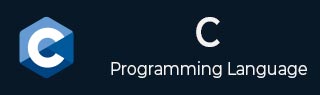
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - puts() function
The C library puts() function is used to write a string to the standard output (usually the console) followed by a newline character. This function simplifies the process of outputting strings, as it automatically appends a newline at the end, unlike printf() which requires explicit inclusion of the newline character.
Syntax
Following is the C library syntax of the puts() function −
int puts(const char *str);
Parameters
This function accepts only a single parameter −
-
str: A pointer to a null-terminated string that you want to print.
Return Value
On success, the puts() function returns a non-negative integer and on failure, it returns EOF (End Of File).
Example 1: Basic Usage
This example shows the basic usage of puts() to print the string "Hello, World!" followed by a newline.
Below is the illustration of the C library puts() function.
#include <stdio.h> int main() { puts("Hello, World!"); return 0; }
Output
The above code produces the following result−
Hello, World!
Example 2: Handling Empty Strings
This example shows that puts() can handle empty strings and will output just a newline when given an empty string.
#include <stdio.h> int main() { // Prints a newline puts(""); puts("Non-empty string after an empty line"); return 0; }
Output
After execution of above code, we get the following result −
Non-empty string after an empty line