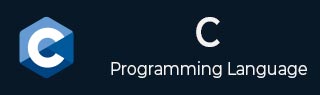
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - free() function
The C stdlib library free() function is used to deallocate or release the memory that was previously allocated by the dynamic memory allocation functions such as calloc(), malloc(), or realloc().
This function helps to prevent memory leaks by allowing programmers to release memory that is no longer needed.
Syntax
Following is the C library syntax of the free() function −
void free(void *ptr)
Parameters
This function accepts a single parameter −
-
*ptr − It represents a pointer to the memory block that need to be freed or deallocate.
Return Value
This function does not returns any values.
Example 1
Let's create a basic c program to demonstrate the use of free() function.
#include <stdio.h> #include <stdlib.h> int main() { //allocate the memory int *ptr = (int*)malloc(sizeof(int)); if (ptr == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } *ptr = 42; printf("Value: %d\n", *ptr); // free the allocated memory free(ptr); // Set 'ptr' NULL to avoid the accidental use ptr = NULL; return 0; }
Output
Following is the output −
Value: 42
Example 2
In this example, we allocate the memory dynamically, and after the allocation we free the memory using free() function.
#include <stdio.h> #include <stdlib.h> int main() { int *ptr; int n = 5; // Dynamically allocate memory using malloc() ptr = (int*)malloc(n * sizeof(int)); // Check if the memory has been successfully allocated if (ptr == NULL) { printf("Memory not allocated.\n"); exit(0); } else { // Memory has been successfully allocated printf("Memory successfully allocated.\n"); // Free the memory free(ptr); printf("Memory successfully freed.\n"); } return 0; }
Output
Following is the output −
Memory successfully allocated. Memory successfully freed.