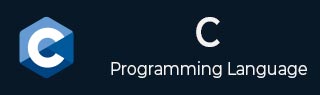
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - fputs() function
The C library int fputs(const char *str, FILE *stream) function writes a string to the specified stream up to but not including the null character.It is commonly used for writing text to files.
Syntax
Following is the C library syntax of the fputs() function −
int fputs(const char *str, FILE *stream);
Parameters
This function accept two parameters −
- const char *str: This is a pointer to the null-terminated string that is to be written to the file.
- FILE *stream: This is a pointer to a FILE object that identifies the stream where the string is to be written. The stream can be an open file or any other standard input/output stream.
Return value
On success, fputs returns a non-negative value. On error, it returns EOF (-1), and the error indicator for the stream is set.
Example 1: Writing a String to a File
This example writes a simple string to a text file.
Below is the illustration of C library fputs() function.
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "w"); if (file == NULL) { perror("Failed to open file"); return 1; } if (fputs("Hello, World!\n", file) == EOF) { perror("Failed to write to file"); fclose(file); return 1; } fclose(file); return 0; }
Output
The above code opens a file named example1.txt for writing, writes "Hello, World!" to it, and then closes the file. −
Hello, World!
Example 2: Writing Multiple Lines to a File
This example writes multiple lines to a file using fputs in a loop.
#include <stdio.h> int main() { FILE *file = fopen("example3.txt", "w"); if (file == NULL) { perror("Failed to open file"); return 1; } const char *lines[] = { "First line\n", "Second line\n", "Third line\n" }; for (int i = 0; i < 3; ++i) { if (fputs(lines[i], file) == EOF) { perror("Failed to write to file"); fclose(file); return 1; } } fclose(file); return 0; }
Output
After execution of above code,it writes three different lines to example3.txt by iterating through an array of strings and using fputs in each iteration.−
First line Second line Third line