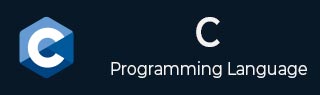
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - fopen() function
The C library function FILE *fopen(const char *filename, const char *mode) opens the filename pointed to, by filename using the given mode. It opens a file and returns a pointer to a FILE object that can be used to access the file.
Syntax
Following is the C library syntax of the fopen() function −
FILE *fopen(const char *filename, const char *mode);
Parameter
This function accepts the following parameters −
- filename: A string representing the name of the file to be opened. This can include an absolute or relative path.
- mode: A string representing the mode in which the file should be opened. Common modes include:
- "r": Open for reading. The file must exist.
- "w": Open for writing. Creates an empty file or truncates an existing file.
- "a": Open for appending. Writes data at the end of the file. Creates the file if it does not exist.
- r+": Open for reading and writing. The file must exist.
- "w+": Open for reading and writing. Creates an empty file or truncates an existing file.
- a+": Open for reading and appending. The file is created if it does not exist.
Return Value
The fopen function returns a FILE pointer if the file is successfully opened. If the file cannot be opened, it returns NULL.
Example 1: Reading a File
This example opens a file named example.txt in read mode and prints its contents to the console character by character.
Below is the illustration of C library fopen() function.
#include <stdio.h> int main() { FILE *file = fopen("example.txt", "r"); if (file == NULL) { perror("Error opening file"); return 1; } char ch; while ((ch = fgetc(file)) != EOF) { putchar(ch); } fclose(file); return 0; }
Output
The above code produces following result−
(Contents of example.txt printed to the console)
Example 2: Appending to a File
Here, we open a file named log.txt in append mode, adds a new log entry to the end of the file, and then closes the file.
#include <stdio.h> int main() { FILE *file = fopen("log.txt", "a"); if (file == NULL) { perror("Error opening file"); return 1; } const char *log_entry = "Log entry: Application started"; fprintf(file, "%s\n", log_entry); fclose(file); return 0; }
Output
After execution of above code, we get the following result −
(log.txt contains the new log entry "Log entry: Application started" appended to any existing content)