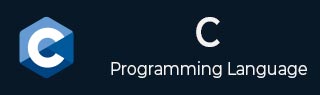
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - atoi() function
The C stdlib library atoi() function is used to convert a numeric string into an integer value.
An integer is a whole number that can be positive or negative, including zero. It can't be fraction, decimal, or percentage, For example, numbers like 1, 2, -5, and -10 are integers.
Syntax
Following is the syntax of the c atoi() function −
int atoi(const char *str)
Parameters
This function accepts a single parameter −
-
str − It is a pointer to a null-terminated sting, which represent the a integer.
Return Value
This function returns an integral number, represents int value. If the input string is not a valid string's number, it returns 0.
Example 1
The following is the basic c example that converts the string value into the integer value using the atoi().
#include <stdlib.h> #include <stdio.h> int main(void) { int val; char *str; str = "1509.10E"; val = atoi(str); printf("integral number = %d", val); }
Output
Following is the output −
integral number = 1509
Example 2
The below c example concatenate two string and then convert the resulting string into a integral number using the atoi().
#include <stdio.h> #include <stdlib.h> #include <string.h> int main() { // Define two strings to concatenate char str1[] = "2023"; char str2[] = "2024"; //calculate the length of string first + second int length = strlen(str1) + strlen(str2) + 1; // Allocate memory for the concatenated string char *concatenated = malloc(length); // check memory allocation if null return 1. if(concatenated == NULL) { printf("Memory allocation failed\n"); return 1; } // Concatenate str1 and str2 strcpy(concatenated, str1); strcat(concatenated, str2); // Convert concatenated string into a integral number. // use the atoi() function int number = atoi(concatenated); printf("The concatenated string is: %s\n", concatenated); printf("The integral number is: %d\n", number); // at the last free the alocated memory free(concatenated); return 0; }
Output
Following is the output −
The concatenated string is: 20232024 The integral number is: 20232024
Example 3
Following is another example of atoi(), here we convert the character string into the integral number.
#include <stdio.h> #include <stdlib.h> #include <string.h> int main () { int res; char str[25]; //define a string strcpy(str, "tutorialspoint India"); //use atoi() function res = atoi(str); printf("String Value = %s\n", str); printf("Integral Number = %d\n", res); return(0); }
Output
Following is the output −
String Value = tutorialspoint India Integral Number = 0