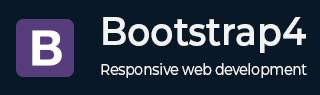
- Bootstrap 4 Tutorial
- Bootstrap 4 - Home
- Bootstrap 4 - Overview
- Bootstrap 4 - Environment Setup
- Bootstrap 4 - Layout
- Bootstrap 4 - Grid System
- Bootstrap 4 - Content
- Bootstrap 4 - Components
- Bootstrap 4 - Utilities
- Differences Between Bootstrap 3 and 4
- Bootstrap 4 Useful Resources
- Bootstrap 4 - Quick Guide
- Bootstrap 4 - Useful Resources
- Bootstrap 4 - Discussion
Bootstrap 4 - Tooltips
Description
Tooltips are useful when you need to describe a link. Tooltip will display a small pop-up box, when you hover the mouse on an element.
Creating a Tooltip
You can add tooltip to an element by adding data-toggle = "tooltip" attribute to it. The title attribute indicates the text of a tooltip.
The following example shows the usage of scrollspy −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href ="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <h2>Tooltip on Link</h2> <p> Hello World!!! Welcome to <a href = "#" data-toggle = "tooltip" title = "Tooltip on link"> Tutorialspoint... </a> <h2>Tooltip on Button</h2> Hello World!!! Welcome to <button type = "button" class = "btn btn-info" data-toggle = "tooltip" data-placement = "top" title = "Tooltip on button"> Tutorialspoint... </button> </p> </div> <script> $(document).ready(function(){ $('[data-toggle = "tooltip"]').tooltip(); }); </script> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
Positioning Tooltips
Tooltip can be displayed in four directions such as top, bottom, left or right side by using the data-placement attribute on the element.
The following example shows positioning of tooltips −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <title>Bootstrap 4 Example</title> </head> <body> <div class = "container"> <br> <h2>Positioning Tooltips</h2> <br> <button type = "button" class = "btn btn-info" data-toggle = "tooltip" data-placement = "top" title = "Tooltip on top">Tooltip on top</button> <button type = "button" class = "btn btn-info" data-toggle = "tooltip" data-placement = "right" title = "Tooltip on right">Tooltip on right</button> <button type = "button" class = "btn btn-info" data-toggle = "tooltip" data-placement = "bottom" title = "Tooltip on bottom">Tooltip on bottom</button> <button type = "button" class = "btn btn-info" data-toggle = "tooltip" data-placement = "left" title = "Tooltip on left">Tooltip on left</button> <script> $(document).ready(function(){ $('[data-toggle = "tooltip"]').tooltip(); }); </script> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity =" sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
bootstrap4_components.htm
Advertisements