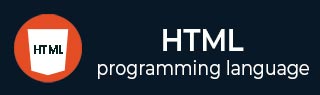
- HTML Tutorial
- HTML - Home
- HTML - History and Evolution
- HTML - Overview
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Formatting
- HTML - Headings
- HTML - Paragraphs
- HTML - Quotations
- HTML - Comments
- HTML - Phrase Tags
- HTML - Meta Tags
- HTML - Style Sheet
- HTML - CSS Classes
- HTML - CSS IDs
- HTML - Images
- HTML - Image Map
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML - Iframes
- HTML - Blocks
- HTML Backgrounds
- HTML - Backgrounds
- HTML Colors
- HTML - Colors
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Code Builder
- HTML References
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - <canvas> Tag
HTML <canvas> tag is used to draw graphics. It designates a section of the webpage where various objects, graphics, animations, and photo compositions can be made using scripts.
This is a new tag included in HTML5. HTML <canvas> tag is only a container for visuals, thus you should use a script if you want to draw them. When working with canvas, it's crucial to make the distinction between frequently conflated ideas like the canvas element and the context of an element. A canvas context is an object with its own set of attributes and rendering strategy. Context may be 2D or 3D. There can only be one context for the canvas element.
Syntax
<canvas> ... </canvas>
Attribute
HTML canvas tag supports Global and Event attributes of HTML. Accept some specipic attributes as well which are listed bellow.
Attribute | Value | Description |
---|---|---|
height | pixels | Specifies the height of the created canvas and the default value is 150. |
width | pixels | Specifies the width of the created canvas and Default value is 300. |
Examples of HTML canvas Tag
Bellow examples will illustrate the usage of canvas tag. Where, when and how to use it to create graphics using canvas tag and how we can style that graphics using CSS.
Creating a Graphics using Canvas tag
Let’s look at the following example, where we are going to draw the circle using the <canvas> tag.
<!DOCTYPE html> <html> <body> <canvas id = "tutorial" height = "200" width = "210" style = "border:2px solid #8E44AD "> </canvas> <script> var x = document.getElementById("tutorial"); var y = x.getContext("2d"); y.beginPath(); y.arc(100, 100, 90, 0, 2 * Math.PI); y.stroke(); </script> </body> </html>
Creating a Texual Graphics
Consider the following example, where we are going to draw the text on the canvas using the canvas tag with strokeText() method.
<!DOCTYPE html> <html> <body> <canvas id="tutorial" width="1000" height="100"></canvas> <script> var x = document.getElementById("tutorial"); var y = x.getContext("2d"); y.font = "60px verdana"; y.strokeStyle = "green"; y.strokeText("TUTORIALSPOINT", 20, 60); </script> </body> </html>
Styling Graphic Element
Following is the example where we are going to make the linear gradient and fill the rectangle with the gradient.
<!DOCTYPE html> <html> <body> <canvas id="tutorial" width="600" height="150" style="border:2px solid #D2B4DE;"> </canvas> <script> var x = document.getElementById("tutorial"); if (x.getContext) { var y = x.getContext("2d"); var gradient = y.createLinearGradient(11, 91, 210, 89); gradient.addColorStop(0, '#DE3163'); gradient.addColorStop(1, '#D5F5E3 '); y.fillStyle = gradient; y.fillRect(11, 12, 570, 120); } </script> </body> </html>
Nested Graphics
In the following example, we are going to use the fillText() method to draw text on the canvas.
<!DOCTYPE html> <html> <body> <canvas id="tutorial" width="500" height="200" style="border:3px solid #27AE60"> </canvas> <script> var x = document.getElementById("tutorial"); var y = x.getContext("2d"); y.font = "bold 35px solid"; y.fillText("TUTORIALSPOINT", 100, 100); </script> </body> </html>
Supported Browsers
Tag | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
canvas | Yes 4.0 | Yes 9.0 | Yes 2.0 | Yes 3.1 | Yes 9.0 |
To Continue Learning Please Login