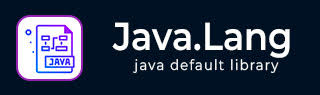
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - String valueOf() Method
Description
The Java String valueOf(boolean b) method is used to get string representation of the passed argument. This method has multiple polymorphic variants. The syntaxes of these methods are given below.
Declaration
Following is the declaration for java.lang.String.valueOf() method
public static String valueOf(boolean b) or public static String valueOf(char c) or public static String valueOf(char[] data) or public static String valueOf(char[] data, int offset, int count) or public static String valueOf(int i) or public static String valueOf(double d) or public static String valueOf(float f or public static String valueOf(long l) or public static String valueOf(Object obj) or
Parameters
data − This is a input to be evaluated.
offset − This is the initial offset into the value of the String.
count − This is the length of the value of the String.
Return Value
if the argument is true, a string equal to "true" is returned else, a string equal to "false" is returned.
Exception
IndexOutOfBoundsException − if offset is negative, or count is negative, or offset+count is larger than data.length.
Getting String Representation of Boolean Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated boolean values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf(true); String str2 = String.valueOf(false); // print the string representation of boolean System.out.println(str1); System.out.println(str2); } }
Let us compile and run the above program, this will produce the following result −
true false
Getting String Representation of Char Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated char values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf('k'); String str2 = String.valueOf('m'); // print the string representation of char System.out.println(str1); System.out.println(str2); } }
Let us compile and run the above program, this will produce the following result −
k m
Getting String Representation of Char Array Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated char array values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // character array chararray1 char[] chararr1 = new char[] { 't', 'u', 't', 's' }; String str1 = String.valueOf(chararr1); // character array chararray2 char[] chararr2 = new char[] { '2', '1', '5' }; String str2 = String.valueOf(chararr2); // prints the string representations System.out.println(str1); System.out.println(str2); } }
Let us compile and run the above program, this will produce the following result −
tuts 215
Getting String Representation of char array with offset Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated char array values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // character array chararray1 with offset 2 char[] chararr1 = new char[] { 't', 'u', 't', 's' }; String str1 = String.valueOf(chararr1, 2, 2); // character array chararray2 with offset 1 char[] chararr2 = new char[] { '2', '1', '5' }; String str2 = String.valueOf(chararr2, 1, 2); // prints the string representations System.out.println(str1); System.out.println(str2); } }
Let us compile and run the above program, this will produce the following result −
ts 15
Getting String Representation of Double Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated double values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf(12.97); String str2 = String.valueOf(Double.MIN_VALUE); String str3 = String.valueOf(Double.MAX_VALUE); // print the string representation of double System.out.println(str1); System.out.println(str2); System.out.println(str3); } }
Let us compile and run the above program, this will produce the following result −
12.97 4.9E-324 1.7976931348623157E308
Getting String Representation of Float Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated float values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf(12.85f); String str2 = String.valueOf(Float.MIN_VALUE); String str3 = String.valueOf(Float.MAX_VALUE); // prints the string representations of float System.out.println(str1); System.out.println(str2); System.out.println(str3); } }
Let us compile and run the above program, this will produce the following result −
12.85 1.4E-45 3.4028235E38
Getting String Representation of Int Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated integer values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf(50); String str2 = String.valueOf(Integer.MIN_VALUE); String str3 = String.valueOf(Integer.MAX_VALUE); // prints the string representations of int System.out.println(str1); System.out.println(str2); System.out.println(str3); } }
Let us compile and run the above program, this will produce the following result −
50 -2147483648 2147483647
Getting String Representation of Long Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated long values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = String.valueOf(432567); String str2 = String.valueOf(Long.MIN_VALUE); String str3 = String.valueOf(Long.MAX_VALUE); // prints the string representations of long System.out.println(str1); System.out.println(str2); System.out.println(str3); } }
Let us compile and run the above program, this will produce the following result −
432567 -9223372036854775808 9223372036854775807
Getting String Representation of Object Example
The following example shows the usage of java.lang.String.valueOf() method. We've evaluated String as Object values and then printed the result.
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "compile online"; Object objVal = str; // returns the string representation of the Object argument System.out.println("Value = " + str.valueOf(objVal)); } }
Let us compile and run the above program, this will produce the following result −
Value = compile online
To Continue Learning Please Login