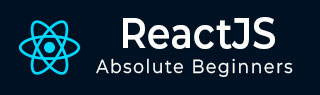
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.update() Method
While working with React we might come across a function called testRenderer.update(). So we will go over what this function performs in simple terms so that even if anyone is new to React, can understand it.
To re-render or refresh the in-memory tree with a new root node, use the testRenderer.update() function. Imagine our React app like a tree, with each component working as a branch. When we use this function, it represents an update to the tree's root.
It is like telling React to repaint the entire tree when we call testRenderer.update(). The new root element serves as the starting point for the refresh. Think of it as a way to represent how React will handle updates. If the new element has the same type and key as the previous one, React updates the tree. If not, it creates a new tree.
Syntax
testRenderer.update(element)
Parameters
element − This is the new root element to be used when updating the in-memory tree. It is the same as giving React a new starting point to refresh our app.
Return Value
The function testRenderer.update(element) does not explicitly return anything. Instead, it takes action by re-rendering the in-memory tree based on the given element. The tree is updated if the new element has the same type and key as the old element. Otherwise, a new tree is mounted.
Examples
Let us see some simple applications to understand the testRenderer.update() method practically.
Example − Basic Update
In this app we will define a simple App component that receives a text prop and displays it in a <div>. Initially, it renders with the text "Hello, React!" and logs the output. Then, it updates the element with a new text prop ("Updated Text!") and logs the updated output.
import React from 'react'; import TestRenderer from 'react-test-renderer'; const App = ({ text }) => <div>{text}</div>; export default App; const testRenderer = TestRenderer.create(<App text="Hello, React!" />); // Initial Output console.log(testRenderer.toJSON()); // Update the element testRenderer.update(<App text="Updated Text!" />); // Updated Output console.log(testRenderer.toJSON());
Output

The initial render shows a div element with the text "Hello, React!" inside. After the update, the div still exists, but the text inside has changed to "Updated Text!".
Example
In this app we will have an App component that displays different text based on the showText prop. Initially, it renders with showText={true}, displaying "Hello, React!" and logs the output. Then, it updates the element with showText={false}, causing it to display "Text Hidden," and logs the updated output.
import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; const App = ({ showText }) => { const textToShow = showText ? "Hello, React!" : "Text Hidden"; return <div>{textToShow}</div>; }; export default App; const testRenderer = TestRenderer.create(<App showText={true} />); // Initial Output console.log(testRenderer.toJSON()); // Update the element conditionally testRenderer.update(<App showText={false} />); // Updated Output console.log(testRenderer.toJSON());
Output

Initially, the div displays "Hello, React!" based on the showText prop being true. After the update, the div now displays "Text Hidden" as the showText prop is set to false.
Example
In this app we will feature an App component with a button that receives buttonText and onClick props. Initially, it renders with "Click me!" and logs the output. Then, it will update the element with "Updated Text!" and a new onClick function, logging the updated output.
import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; const App = ({ buttonText, onClick }) => ( <div> <button onClick={onClick}>{buttonText}</button> </div> ); export default App; const testRenderer = TestRenderer.create( <App buttonText="Click me!" onClick={() => console.log("Button Clicked!")} /> ); // Initial Output console.log(testRenderer.toJSON()); // Update the element dynamically testRenderer.update( <App buttonText="Updated Text!" onClick={() => console.log("Updated Click!")} /> ); // Updated Output console.log(testRenderer.toJSON());
Output

The initial render includes a div with a button saying "Click me!" and an onClick function. After the update, the button's text changes to "Updated Text!" while the onClick function remains the same.
Summary
testRenderer.update() is a React tool for managing changes in our app. It is like giving our app a refresh button, which allows it to respond to new information or user activities. Whether it is a small change or a complete change, this function maintains our React app active and responsive.
So we have created some testing for the apps using this function. These examples above show how the testRenderer.update(element) method can be used to modify React components and see the changes in the rendered output.
To Continue Learning Please Login