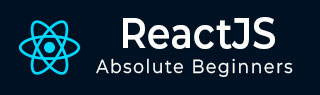
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.toTree() Method
testRenderer.toTree() is a testing function which records and represents a detailed image of the rendered structure, similar to taking a snapshot of how things look in a test scenario. This function provides more information than other methods, like toJSON(), and includes user-written components. In general, we will use it while creating our own testing tools or when we want a very specific view of the created items during programming testing.
This can be useful when testing things in computer programs, especially when we want to check the sections that other people have created. It is similar to looking at an in-depth map to understand all the different parts of an application. So, testRenderer.toTree() allows us to view what is going on in our test in a detailed way.
Syntax
testRenderer.toTree()
Parameters
testRenderer.toTree() does not need any parameters. It is like taking a picture of what is happening in a test, and it does not need any extra information to do that.
Return Value
The testRenderer.toTree() function, returns an object that displays the rendered tree.
Examples
Example − In Basic Component
So first we are going to create a simple app in which we will use the testRenderer.toTree() function. This app will be like taking a picture of a simple message in React. The message says, "Hello, React!" and it is inside a box. The function testRenderer.toTree() helps us see how this message and box are put together.
import React from 'react'; import TestRenderer from 'react-test-renderer'; // Simple functional component const MyComponent = () => <div><h1>Hello, React! </h1></div>; export default MyComponent; // Creating a test renderer const testRenderer = TestRenderer.create(<MyComponent />); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
Output

Example − In Props and State
In the second app we are going to create a Counter app. Suppose that the counter is like the numbers on a scoreboard. This app is like taking a snapshot of that counter in React. We can increase the count by clicking a button. The function testRenderer.toTree() shows us how this counter and button are set up.
import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; // Component with props and state const Counter = ({ initialCount }) => { const [count, setCount] = useState(initialCount); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter; // Creating a test renderer with props const testRenderer = TestRenderer.create(<Counter initialCount={5} />); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
Output

Example − Component with Children
Lastly we will create a component in which we will have children. It will be like capturing a family photo in React. There is a parent component in which the message "Parent Component" will be displayed and a child component in which a message is "I'm a child" message will be shown. The testRenderer.toTree() function lets us see how these parent and child components are arranged.
import React from 'react'; import TestRenderer from 'react-test-renderer'; // Component with children const ParentComponent = ({ children }) => ( <div> <h2>Parent Component</h2> {children} </div> ); export default ParentComponent; // Child component const ChildComponent = () => <p>I'm a child!</p>; // test renderer with nested components const testRenderer = TestRenderer.create( <ParentComponent> <ChildComponent /> </ParentComponent> ); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
Output

When calling testRenderer.toTree(), the output for each of the three apps looks like a detailed representation of the generated tree. The output will be an object with information on the components, their props, and the virtual DOM structure.
Summary
So in this tutorial we have learned about the testRenderer.toTree() method. We explored three simple React applications and used the testRenderer.toTree() function to take a detailed snapshot of how they were rendered. Each app represented a different scenario. It helps us understand the structure of our components and how they interact with each other.
To Continue Learning Please Login