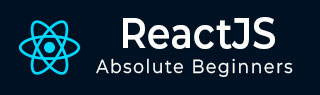
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.root Property
"testRenderer.root" is a programming command. It is like a key that unlocks and grants entry to the main or upper level of a testing structure. This key allows programmers to evaluate and test various elements or parts of an application.
It is like the primary branch of a tree, directing us through the branches and leaves that represent different elements in the app. In simple terms, "testRenderer.root" allows developers to review and ensure that everything in their code is operating properly.
Syntax
testRenderer.root
Parameters
This method does not accept any argument.
Return Value
This method will return the main "test instance" or the starting point for checking and understanding different parts of our code.
Examples
Example − Basic Component Test
The will have a simple React component (MyComponent.js) and a corresponding test file (MyComponent.test.js). This is a basic React functional component named MyComponent. It does not take any props and simply returns a JSX element, rendering a <div> with the text "Hello, World!". And MyComponent.test.js is a test file for MyComponent. It uses the react-test-renderer library, which is a React package for rendering components in tests. The test function is used to define a test case, checking if MyComponent renders correctly.
MyComponent.js
import React from 'react'; const MyComponent = () => { return <div>Hello, World!</div>; }; export default MyComponent; // MyComponent.test.js import React from 'react'; import TestRenderer from 'react-test-renderer'; import MyComponent from './MyComponent'; test('renders MyComponent correctly', () => { const testRenderer = TestRenderer.create(<MyComponent />); const root = testRenderer.root; // Perform assertions using root expect(root.findByType('div').props.children).toBe('Hello, World!'); });
Output

So, it performs an assertion, checking if the text content of the <div> element inside MyComponent is "Hello, World!".
Example − Testing With Props
In this app the code consists of a React component (Greeting.js) and a corresponding test file (Greeting.test.js). Greeting.js is a React functional component named Greeting. It takes a prop name and uses it to dynamically render a greeting message in a <div> element. The message says "Hello, " followed by the value of the name prop. Greeting.test.js is a test file for the Greeting component. It uses the react-test-renderer library to create a test renderer and render the Greeting component with the prop name set to "Ankit".
Greeting.js
import React from 'react'; const Greeting = ({ name }) => { return <div>Hello, {name}!</div>; }; export default Greeting;
Greeting.test.js
import React from 'react'; import TestRenderer from 'react-test-renderer'; import Greeting from './Greeting'; test('renders Greeting correctly with props', () => { const testRenderer = TestRenderer.create(<Greeting name="Ankit" />); const root = testRenderer.root; // Perform assertions using root expect(root.findByType('div').props.children).toBe('Hello, Ankit!'); });
Output

Example
In this app we will have a React component (Counter.js) and a corresponding test file (Counter.test.js). Counter.js file is a React functional component. It maintains a state variable count using the useState hook, initialized to 0. The component renders a <div> containing a <p> element displaying the current count and a <button> that, when clicked, calls the increment function and updates the count. Counter.test.js file is a test file for the Counter component. The test case is created to check whether the count is correctly incremented when the button is clicked.
Counter.js
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => setCount(count + 1); return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }; export default Counter;
Counter.test.js
import React from 'react'; import TestRenderer from 'react-test-renderer'; import Counter from './Counter'; test('increments count when the button is clicked', () => { const testRenderer = TestRenderer.create(<Counter />); const root = testRenderer.root; // Check initial count expect(root.findByType('p').props.children).toBe('Count: 0'); // Simulate button click root.findByType('button').props.onClick(); // Check if count is incremented expect(root.findByType('p').props.children).toBe('Count: 1'); });
Output

Summary
testRenderer.root is a method used in testing React components. When using a testing library like react-test-renderer, this method provides access to the root or top-level instance of the rendered component tree. It allows testers to make assertions and perform tests on different parts of the component. So we have seen the usage of this method and created three different apps to understand this concept practically.
To Continue Learning Please Login