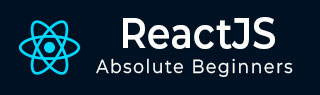
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.props Property
When it comes to web development and creating user interfaces, there is a concept known as testInstance.props. Consider it to be a set of instructions for a certain element on a website.
Props are messages that a parent component in a web application sends to its child components. Suppose we have a button on a website and want to tell it to be small. In the programming terms, we will say something like <Button size="small" />. Size is a prop in this case, and its value is 'small.'
When we come across testInstance.props, it is as if we are looking at all of the messages or instructions that have been delivered to certain parts of the website during testing. For example, if we have a <Button size="small" /> component, its testInstance.props would be {size:'small'}. It is made up mainly of these messages or characteristics.
Syntax
testInstance.props
Parameters
The testInstance.props does not accept any parameters.
Return Value
The testInstance.props property returns a collection of instructions associated with a particular element in a web application during testing.
Examples
Example − Button Component
This is a simple React component called Button. It is build to create a button with customizable properties like font size, background color, and label. The component logs its properties during testing for debugging purposes. If specific styles or labels are not provided through props, default values are used to ensure a visually appealing button. So the code for this app is given below −
import React from 'react'; const Button = (props) => { const { fontSize, backgroundColor, label } = props; console.log('Button Props:', props); const buttonStyle = { fontSize: fontSize || '16px', backgroundColor: backgroundColor || 'blue', padding: '10px 15px', borderRadius: '5px', cursor: 'pointer', color: '#fff', // Text color }; const buttonLabel = label || 'Click me'; return ( <button style={buttonStyle}> {buttonLabel} </button> ); }; export default Button;
Output

Example − Image Component
In this example, the App component renders the Image component with a set of image props like src, alt, and width. We can replace the placeholder values with the actual URL, alt text, and width of the desired image. The console.log statement in the Image component will log these props during testing. So the code is as follows −
Image.js
import React from 'react'; const Image = (props) => { const { src, alt, width } = props; console.log('Image Props:', props); // Log the props here return <img src={src} alt={alt} style={{ width }} />; }; export default Image;
App.js
import React from 'react'; import Image from './Image'; const App = () => { const imageProps = { src: 'https://www.example.com/picphoto.jpg', // Replace with the actual image URL alt: 'Sample Image', width: '300px', }; return ( <div> <h1>Image Component Example</h1> <Image {...imageProps} /> </div> ); }; export default App;
Output

Example − Input Component
In this example, the App component renders the Input component with a set of input props like placeholder and maxLength. The console.log statement in the Input component will log these props during testing. And the code for this component is given below −
Input.js
import React from 'react'; const Input = (props) => { const { placeholder, maxLength } = props; console.log('Input Props:', props); // Log the props here return <input type="text" placeholder={placeholder} maxLength={maxLength} />; }; export default Input;
App.js
import React from 'react'; import Input from './Input'; const App = () => { const inputProps = { placeholder: 'Enter your text', maxLength: 50, }; return ( <div> <h1>Input Component Example</h1> <Input {...inputProps} /> </div> ); }; export default App;
Output

Summary
testInstance.props is a property that allows developers to view and understand the properties or instructions assigned to certain elements on a website during testing. It is like looking at a cheat sheet that explains how each component of our web application should work. By understanding this principle, developers can ensure that their websites function properly and give users an excellent experience.
To Continue Learning Please Login