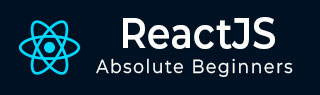
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.findByProps() Method
testInstance.findByProps(props) is a testing function. testInstance most likely refers to a testing instance or object in a software testing environment. The simple fact that findByProps(props) is a component shows that we are searching for something specific within the test instance. The term "props" refers to properties, which are attributes of the item we are looking for.
This code finds a single test object with specific properties. It is like looking for a specific item in many. If there is only one match, it returns that instance. If not, an error message is displayed.
Syntax
testInstance.findByProps(props)
Parameters
props − The term "props" refers to properties, which are attributes of the item we are looking for.
Return Value
This code looks for a single test object with specific features.It is like looking for an object. If there is just one match, that instance is returned. If this is not the case, an error message is displayed.
Examples
Example − Basic Component Test
Creating React apps using the testInstance.findByProps(props) method involves writing test cases to find specific elements with certain properties in our React components. Below is a simple code for this app −
MyComponent.js
import React from 'react'; const MyComponent = ({ title }) => ( <div> <h1>{title}</h1> <p>Hello, this is a simple component.</p> </div> ); export default MyComponent;
MyComponent.test.js
import React from 'react'; import { render } from '@testing-library/react'; import MyComponent from './MyComponent'; test('finds the title using findByProps', () => { const { findByProps } = render(<MyComponent title="My App" />); const titleElement = findByProps({ title: 'My App' }); expect(titleElement).toBeInTheDocument(); });
Output

Example − List Component Test
Now we will create a React component named ListComponent. It takes a prop item which is an array. It renders an unordered list (<ul>) with list items (<li>) generated from the elements of the items array.
And then we will create ListComponent.test.js for the ListComponent component. It uses the render function from the @testing-library/react library to render the ListComponent with prop items. The test function is checking that the list item with the text 'Item 2' is present in the rendered component using findByProps.
ListComponent.js
import React from 'react'; const ListComponent = ({ items }) => ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); export default ListComponent;
ListComponent.test.js
import React from 'react'; import { render } from '@testing-library/react'; import ListComponent from './ListComponent'; test('finds the list item using findByProps', () => { const items = ['Item 1', 'Item 2', 'Item 3']; const { findByProps } = render(<ListComponent items={items} />); const listItem = findByProps({ children: 'Item 2' }); expect(listItem).toBeInTheDocument(); });
Output

This code tests the rendering of a ListComponent with a list of items and checks if a specific list item ('Item 2') is present in the component with the help of findByProps method.
Example − Form Component Test
This time we will create a React component named FormComponent. It consists of a form with an input field. The input field is controlled using the useState hook to manage the inputValue. The label has the htmlFor attribute, with the input field using the same id.
And then we will create a test file (FormComponent.test.js) for the FormComponent component. The test checks that the input field starts with an empty value ('') and asserts that it is initially present in the document. The fireEvent.change simulates a user typing 'Hello, World!' into the input field. It then uses findByProps to search for an input field with the updated value and asserts that it is present in the document.
FormComponent.js
import React, { useState } from 'react'; const FormComponent = () => { const [inputValue, setInputValue] = useState(''); return ( <form> <label htmlFor="inputField">Type something:</label> <input type="text" id="inputField" value={inputValue} onChange={(e) => setInputValue(e.target.value)} /> </form> ); }; export default FormComponent;
FormComponent.test.js
import React from 'react'; import { render, fireEvent } from '@testing-library/react'; import FormComponent from './FormComponent'; test('finds the input value using findByProps', async () => { const { findByProps } = render(<FormComponent />); const inputElement = findByProps({ value: '' }); expect(inputElement).toBeInTheDocument(); fireEvent.change(inputElement, { target: { value: 'Hello, World!' } }); const updatedInputElement = findByProps({ value: 'Hello, World!' }); expect(updatedInputElement).toBeInTheDocument(); });
This code tests the rendering and user interaction of a FormComponent by checking if the input field updates correctly when a user types into it.
Summary
So the testInstance.findByProps(props) helps us to find an object with specific properties during a test. It is successful if it discovers exactly one match. Otherwise, an error is sent. We have seen different applications and cases in which we can use this function for testing React apps.
To Continue Learning Please Login