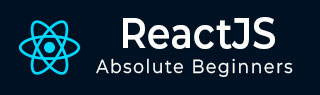
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.find() Method
The term "testInstance" is not a defined React concept, but it is often used typically when testing React components. It refers to the instance of a component generated when it is rendered in a testing environment, allowing us to execute tests and make assertions about its behavior.
In programming, we may need to use the find() function to locate a specific test instance. Let's get started and go over how to use it in this tutorial.
The find() function acts as a detective in finding something among a group of objects. It works by comparing each object to a criteria set by another method called test(). The objective is to find only one object that meets the requirement.
Syntax
testInstance.find(test)
Parameters
testInstance − This is the group of objects we want to search through.
test − This is a special function we create. It checks each object to see if it meets certain criteria. If it returns true for one object, if the object is found.
Return Value
The find() function looks at each object in testInstance. For each object, it runs the test() function. If test() returns true for exactly one object, that object is the result. If test() does not return true for exactly one object, an error occurs.
Examples
Example − Button Component
In the example we will have a Button component and its corresponding test file. So we can make assertions based on the expected behavior after clicking the button. Let us say we want to check if a certain function (mockFunction) is called when the button is clicked. The code is as follows −
Button.js
import React from 'react'; const Button = ({ onClick, label }) => ( <button onClick={onClick} data-testid="test-button"> {label} </button> ); export default Button;
Button.test.js
import React from 'react'; import { render, fireEvent, screen } from '@testing-library/react'; import Button from './Button'; test('Button click works', () => { // Mock function to check if it is called const mockFunction = jest.fn(); render(<Button onClick={mockFunction} label="Click me" />); const buttonInstance = screen.getByTestId('test-button'); fireEvent.click(buttonInstance); expect(mockFunction).toHaveBeenCalledTimes(1); });
Output

Example − List Component
In this example the test is for the List component, we have to make assertions about the rendered items in the list. Let us say we want to check if the list contains specific items in the correct order. The toHaveTextContent function is used to check that the displayed listInstance contains the requested text content.
List.js
import React from 'react'; const List = ({ items }) => ( <ul data-testid="test-list"> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); export default List;
List.test.js
import React from 'react'; import { render, screen } from '@testing-library/react'; import List from './List'; test('List renders items', () => { const items = ['Item 1', 'Item 2', 'Item 3']; render(<List items={items} />); const listInstance = screen.getByTestId('test-list'); expect(listInstance).toHaveTextContent('Item 1'); expect(listInstance).toHaveTextContent('Item 2'); expect(listInstance).toHaveTextContent('Item 3'); });
Output

Example − Form Component
In this app we will have a Form Component Form.js and its respective test file Form.test.js. In the test for the Form component, after simulating a change event on the input, we want to check if the value of the input has been updated accordingly. So the code is as follows −
Form.js
import React, { useState } from 'react'; const Form = () => { const [inputValue, setInputValue] = useState(''); const handleChange = (e) => { setInputValue(e.target.value); }; return ( <form data-testid="test-form"> <input type="text" value={inputValue} onChange={handleChange} data-testid="test-input" /> </form> ); }; export default Form;
Form.test.js
import React from 'react'; import { render, fireEvent, screen } from '@testing-library/react'; import Form from './Form'; test('Form input changes value', () => { render(<Form />); const inputInstance = screen.getByTestId('test-input'); fireEvent.change(inputInstance, { target: { value: 'New Value' } }); expect(inputInstance.value).toBe('New Value'); });
Output
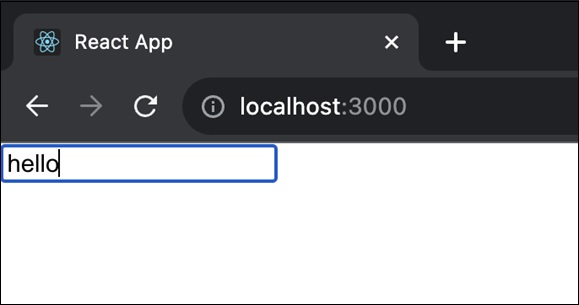
Summary
So, the find() function allows us to search through a collection of objects with the help of a special condition-checking function. Now we have gained in-depth knowledge about the testInstance.find() method by creating three different applications.
To Continue Learning Please Login