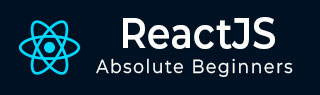
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.children Property
The React.js library is all about dividing the program into many components. Each component has a unique lifecycle. React includes some built-in methods that we can override at various points of the component's life-cycle.
So in this tutorial, we will learn how to use the testInstance.children property. The testInstance.children property is used to retrieve the test instance's children.
The "testInstance.children" property is a programming tool for retrieving the children or smaller components connected with a specific test instance. A test instance represents a main entity in the coding language, and by adding ".children" to it, developers can access and interact with the individual instances nested in it. It is like looking into a larger container to find and interact with one of the pieces located within.
This characteristic is useful for organizing and managing information because it allows programmers to focus on and manipulate small details within a larger context, resulting in more efficient and precise coding techniques.
Syntax
testInstance.children
Return Value
The "testInstance.children" property returns a collection or list of the child elements related with the specified test instance.
Examples
Below are some apps using import React from 'react'; and import TestRenderer from 'react-test-renderer'; with the usage of the TestRenderer.children property −
Example − Task Manager App
This app is like a digital to-do list. It uses a special tool called TestRenderer to create a virtual representation of the app. Then, it checks and shows the parts or "children" inside the app using the TestRenderer.children property.
import React from 'react'; import TestRenderer from 'react-test-renderer'; const TaskManagerApp = () => { function showChildren() { const renderer = TestRenderer.create( <div> Task Manager App <div>Task Renderer</div> </div> ); const myChildren = renderer.root; console.log(myChildren.children); } showChildren(); return <> <h1>This is an example of testInstance.children</h1> </>; }; export default TaskManagerApp;
Output

Example − Shopping Cart App
Imagine this app as a simple online shopping cart. It uses TestRenderer, a special tool, to create a virtual version of the app. Then, it checks and shows the parts or "children" inside the app using the TestRenderer.children property.
import React from 'react'; import TestRenderer from 'react-test-renderer'; const ShoppingCartApp = () => { function showChildren() { const renderer = TestRenderer.create( <div> Shopping Cart App <div>Cart Renderer</div> </div> ); const myChildren = renderer.root; console.log(myChildren.children); } showChildren(); return <><h1>This is an example of testInstance.children</h1></>; }; export default ShoppingCartApp;
Output

Example − Drawing Canvas App
This app is like a digital canvas where we can draw pictures. It uses a special tool, TestRenderer, to create a virtual version of the app. Then, it checks and shows the parts or "children" inside the app using the TestRenderer.children property.
import React from 'react'; import TestRenderer from 'react-test-renderer'; const DrawingCanvasApp = () => { function showChildren() { const renderer = TestRenderer.create( <div> Drawing Canvas App <div>Canvas Renderer</div> </div> ); const myChildren = renderer.root; console.log(myChildren.children); } showChildren(); return <><h1>This is an example of testInstance.children</h1></>; }; export default DrawingCanvasApp;
Output

Summary
Finally, testInstance.children is a coding command designed to handle multiple elements within a program. It functions like a magnifying glass, allowing developers to focus on specific areas of their code. Understanding these kinds of commands is important for anyone entering into the world of programming.
To Continue Learning Please Login