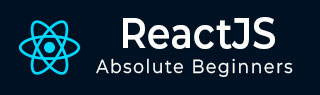
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.parent Property
In programming, especially in the context of testing, the testInstance.parent property is a way to refer to the higher-level or enclosing structure that surrounds a specific test instance. Think of it as finding the 'container' of a particular piece of code.
For example, if we have a test suite that contains multiple test cases, each test case is like a child, and the test suite is the parent. So, if we say testInstance.parent, we are essentially asking, "What is the group or collection that this specific test case belongs to!"
This property is useful for organizing and understanding the hierarchy of our tests. It helps in managing and running tests in groups, making it easier to handle and analyze results.
It is like putting our belongings in different boxes. Each box (test case) has its own content, but the bigger box (test suite) holds them all together. So, testInstance.parent helps us figure out which big box contains the specific small box we are interested in.
Syntax
testInstance.parent
Return Value
The testInstance.parent typically returns the parent or the higher-level container of the specific test instance. It tells us what group or collection the current test instance belongs to.
Examples
Example − Basic Parent-Child Relationship
In this React app we will have three components: ParentComponent, ChildComponent, and the main App component. The ParentComponent renders a div with an <h2> heading, and it wraps the ChildComponent within the ParentContext.Provider, giving a value of 'Parent' to the context. And the ChildComponent uses React.useContext to access the value from the ParentContext and logs it. And it renders a div with an <h3> heading. And lastly the main App component renders a div with an <h1> heading and includes the ParentComponent. So the code for this complete app is as follows −
ParentComponent.js
import React from 'react'; import ChildComponent from './ChildComponent'; // Create a context const ParentContext = React.createContext(); const ParentComponent = () => { return ( <ParentContext.Provider value={'Parent'}> <div> <h2>Parent Component</h2> <ChildComponent /> </div> </ParentContext.Provider> ); }; export default ParentComponent;
ChildComponent.js
import React from 'react'; // Import the context import ParentContext from './ParentComponent'; const ChildComponent = () => { // Use the context const parent = React.useContext(ParentContext); console.log('Parent of ChildComponent:', parent); return ( <div> <h3>Child Component</h3> </div> ); }; export default ChildComponent;
App.js
import React from 'react'; import ParentComponent from './ParentComponent'; const App = () => { return ( <div> <h1>React App</h1> <ParentComponent /> </div> ); }; export default App;
Output

Example − Nested Components
In this app we will see a parent-child-grandchild relationship between components, with each component logging its parent context. The rendered output will show a hierarchy of components with their own headings, and in the console, we will see the logged parent context for each child and grandchild component. So the complete app is given below −
ParentComponent.js
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { return ( <div> <h2>Parent Component</h2> <ChildComponent /> </div> ); }; export default ParentComponent;
ChildComponent.js
import React from 'react'; import GrandchildComponent from './GrandchildComponent'; const ChildComponent = () => { const parent = React.useContext(ParentContext); console.log('Parent of ChildComponent:', parent); return ( <div> <h3>Child Component</h3> <GrandchildComponent /> </div> ); }; export default ChildComponent;
GrandchildComponent.js
import React from 'react'; const GrandchildComponent = () => { const parent = React.useContext(ParentContext); console.log('Parent of GrandchildComponent:', parent); return ( <div> <h4>Grandchild Component</h4> </div> ); }; export default GrandchildComponent;
App.js
import React from 'react'; import ParentComponent from './ParentComponent'; const App = () => { return ( <div> <h1>React App 2</h1> <ParentComponent /> </div> ); }; export default App;
Output

Example − Dynamic Component Rendering
This React app has a dynamic component structure that uses context to share data. The ParentComponent creates a context called ParentContext with the value 'Parent' and functions as a provider for its children. The useContext hook is used by the DynamicComponent to get and log the context value. This app will dynamically render components with names. The code is given below −
ParentComponent.js
import React from 'react'; // Create a context const ParentContext = React.createContext(); const ParentComponent = ({ children }) => { return ( <ParentContext.Provider value={'Parent'}> <div> <h2>Parent Component</h2> {children} </div> </ParentContext.Provider> ); }; export { ParentComponent, ParentContext };
DynamicComponent.js
import React from 'react'; import { ParentContext } from './ParentComponent'; const DynamicComponent = ({ name }) => { const parent = React.useContext(ParentContext); console.log(`Parent of ${name} Component:`, parent); return ( <div> <h3>{name} Component</h3> </div> ); }; export default DynamicComponent;
App.js
import React from 'react'; import DynamicComponent from './DynamicComponent'; const App = () => { const componentsToRender = ['First', 'Second', 'Third']; return ( <div> <h1>React App</h1> {componentsToRender.map((component, index) => ( <DynamicComponent key={index} name={component} /> ))} </div> ); }; export default App;
Output

Summary
In programming, mainly in testing, the testInstance.parent property refers to the structure that wraps around a single test. It is like finding the 'container' of a piece of code. So, using testInstance.parent helps us figure out which big box contains the specific small box we are interested in. As we have seen different examples of this property to get the practical exposure of it.
To Continue Learning Please Login