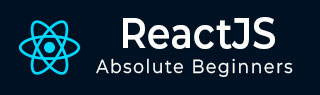
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.findAllByProps() Method
The testInstance.findAllByProps() method is used to find all the descendant test instances that have some properties. In simple terms we can say that it helps us locate and get all the elements in a test that match particular characteristics.
If we want to be more specific and find instances with particular properties, we can use testInstance.findAllByProps(props). This means we are searching for test instances that have the specified properties (attributes or features). This method is a tool for finding and collecting elements in a test based on their properties. It is like a search function for test components.
Syntax
testInstance.findAllByProps(props)
Parameters
props − This is the input we provide to the function. It shows the properties or characteristics we want to find in the test instances. These can be specific attributes or features that we are looking for.
Return Value
The function will return a collection of test instances that match the particular properties. It gives us a list of elements in the test that have the characteristics we are searching for.
Examples
Example − Basic Component with Props
We will create a simple React component and its corresponding test file. This file defines a basic React component named SimpleComponent. It takes a prop text and renders it inside a div element. In the test file, a test case is written to make sure that the SimpleComponent renders the provided text correctly. It uses findAllByProps to find elements with specific props. So the code for this app is as follows −
SimpleComponent.js
import React from 'react'; const SimpleComponent = ({ text }) => { return <div>{text}</div>; }; export default SimpleComponent;
SimpleComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import SimpleComponent from './SimpleComponent'; test('renders text correctly', () => { const component = create(<SimpleComponent text="Hello, World!" />); const instance = component.root; const elements = instance.findAllByProps({ text: 'Hello, World!' }); expect(elements.length).toBe(1); });
Output

Example − List Component with props
Now let us create a ListComponent and its corresponding test file. In this file, a React component named ListComponent will be defined. It will take a prop items and renders an unordered list containing list items for each item in the array. This test file contains a test case that checks if the ListComponent renders the list items correctly.The code for this example is given below −
ListComponent.js
import React from 'react'; const ListComponent = ({ items }) => { return ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); }; export default ListComponent;
ListComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import ListComponent from './ListComponent'; test('renders list items correctly', () => { const items = ['Item 1', 'Item 2', 'Item 3']; const component = create(<ListComponent items={items} />); const instance = component.root; const elements = instance.findAllByProps({ children: items }); expect(elements.length).toBe(items.length); });
Output

Example − Conditional Rendering Component
In this React app we will create a simple ToggleComponent that will have a button to toggle the visibility of a paragraph. The component will also have a corresponding test file, ToggleComponent.test.js, which uses the react-test-renderer library to test the conditional rendering behavior.
ToggleComponent.js
import React, { useState } from 'react'; const ToggleComponent = () => { const [isToggled, setToggle] = useState(false); return ( <div> <button onClick={() => setToggle(!isToggled)}>Toggle</button> {isToggled && <p>This is visible when toggled!</p>} </div> ); }; export default ToggleComponent;
ToggleComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import ToggleComponent from './ToggleComponent'; test('renders paragraph conditionally', () => { // Create a test instance const component = create(<ToggleComponent />); const instance = component.root; // Find elements in the component with props const elements = instance.findAllByProps({ children: 'This is visible when toggled!' }); expect(elements.length).toBe(0); instance.findByType('button').props.onClick(); const updatedElements = instance.findAllByProps({ children: 'This is visible when toggled!' }); expect(updatedElements.length).toBe(1); });
Output

This example shows a simple way to test a React component with conditional rendering. The test case makes sure that the paragraph is initially not visible and becomes visible when the button is clicked.
Summary
The testInstance.findAllByProps() function is commonly used in testing libraries for React to locate and interact with components based on their properties. This function makes it easier to test and validate specific behaviors in our application. This function is used to find all descendant test instances with specific properties. So we have seen different scenarios in which we can use this method to test our apps.
To Continue Learning Please Login