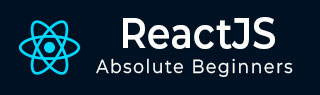
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - scryRenderedDOMComponentsWithTag()
The scryRenderedDOMComponentsWithTag() function is a React programming tool for finding and gathering information about specific elements of a web page. It is simply a method for computer programmers to search for specific items on a website. This scryRenderedDOMComponentsWithTag() function searches for items with a specific tag name, like buttons, images, or paragraphs.
So, when we use scryRenderedDOMComponentsWithTag(tree, tagName), we are instructing the system to search the 'tree' for any items that have the given 'tagName'.
Suppose we have a large, complex webpage and want to find all of the buttons on it. Instead of manually searching, we can use scryRenderedDOMComponentsWithTag() to handle all of the work for us.
Syntax
scryRenderedDOMComponentsWithTag( tree, tagName )
Parameters
The function takes two important things into account: the tree and the tagName.
tree − The tree is like a map of the webpage, showing all the different parts and how they connect.
tagName − The tagName is simply the name of the type of element we are interested in, like div or p.
Return Value
The scryRenderedDOMComponentsWithTag() function returns a list of all the DOM (Document Object Model) elements in the rendered tree that match the specified tag name.
Below are some simple React apps using the scryRenderedDOMComponentsWithTag() method, along with a brief explanation for each −
Examples
Example − Button Counter App
This app displays a button and a count. So when we click the button it will increment the count. To test scryRenderedDOMComponentsWithTag() in React, we will normally use a testing library like Jest or the React Testing Library. So below is the code for both app and testing −
ButtonCounter.js
import React, { useState } from 'react'; import './App.css'; const ButtonCounter = () => { const [count, setCount] = useState(0); return ( <div className='App'> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default ButtonCounter;
Test Code for Button Counter App
import React from 'react'; import { render, scryRenderedDOMComponentsWithTag } from '@testing-library/react'; import ButtonCounter from './ButtonCounter'; test('renders ButtonCounter component', () => { const { container } = render(<ButtonCounter />); const buttons = scryRenderedDOMComponentsWithTag(container, 'button'); expect(buttons.length).toBe(1); });
Output

Example − Todo List App
The application allows us to create and show a to-do list. And we can add a new todo to the list by entering it in the text box and clicking the "Add Todo" button. So below is the code for React App and also the testing for the particular app we have created −
TodoList.js
import React, { useState } from 'react'; import './App.css' const TodoList = () => { const [todos, setTodos] = useState([]); const [newTodo, setNewTodo] = useState(''); const addTodo = () => { setTodos([...todos, newTodo]); setNewTodo(''); }; return ( <div className='App'> <ul> {todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> <input type="text" value={newTodo} onChange={(e) => setNewTodo(e.target.value)} /> <button onClick={addTodo}>Add Todo</button> </div> ); }; export default TodoList;
Test Code for Todo List App
import React from 'react'; import { render, scryRenderedDOMComponentsWithTag } from '@testing-library/react'; import TodoList from './TodoList'; test('renders TodoList component', () => { const { container } = render(<TodoList />); const buttons = scryRenderedDOMComponentsWithTag(container, 'button'); const input = scryRenderedDOMComponentsWithTag(container, 'input'); expect(buttons.length).toBe(1); expect(input.length).toBe(1); });
Output

Example − Color Picker App
This app allows us to select a color from three options: Red, Green, and Blue. The selected color is displayed below the buttons. So the code for both app and its testing is as follows −
ColorPicker.js
import React, { useState } from 'react'; const ColorPicker = () => { const [selectedColor, setSelectedColor] = useState(''); const handleColorChange = (color) => { setSelectedColor(color); }; return ( <div> <p>Selected Color: {selectedColor}</p> <button onClick={() => handleColorChange('Red')}>Red</button> <button onClick={() => handleColorChange('Green')}>Green</button> <button onClick={() => handleColorChange('Blue')}>Blue</button> </div> ); }; export default ColorPicker;
Test Code for Color Picker App
import React from 'react'; import { render, scryRenderedDOMComponentsWithTag } from '@testing-library/react'; import ColorPicker from './ColorPicker'; test('renders ColorPicker component', () => { const { container } = render(<ColorPicker />); const buttons = scryRenderedDOMComponentsWithTag(container, 'button'); const paragraphs = scryRenderedDOMComponentsWithTag(container, 'p'); expect(buttons.length).toBe(3); expect(paragraphs.length).toBe(1); });
Output

Description for each test Cases above
In the above test cases −
To render each component, we use the render function from the React Testing Library.
scryRenderedDOMComponentsWithTag is then used to locate items in the rendered component that have certain HTML tags.
Finally, assertions are made to confirm that there are the expected number of elements with the given tag.
Install the necessary testing libraries (@testing-library/react and @testing-library/jest-dom) −
npm install --save-dev @testing-library/react @testing-library/jest-dom
Summary
The scryRenderedDOMComponentsWithTag() function is a method used in React Testing Library for finding and retrieving DOM elements within a rendered React component. This function takes two parameters: the rendered component (container) and the HTML tag name we want to search for.
To Continue Learning Please Login