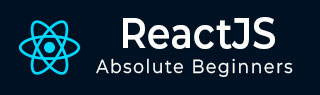
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - renderIntoDocument()
React is a powerful library for creating user interfaces in the world of web development. Rendering React elements into the Document Object Model (DOM) is an important process. In this tutorial, we will look at the renderIntoDocument() function and how it works in simple words.
The renderIntoDocument() function is part of React and is responsible for displaying a React element on a web page. It displays the element in a separate space in the document, separate from the rest of the content.
The primary goal of renderIntoDocument() is to make a React component visible on the web page. It is particularly helpful when we want to display React content in a separate area from the rest of the document.
Syntax
renderIntoDocument(element)
Parameters
element − It is a React element which is rendered into a detached DOM node in the document.
Return Value
In React, the renderIntoDocument() function does not immediately return anything. However, it performs a task: it converts a React element into a separate DOM node in the document. It means that the specified React element is displayed in a specific area of the webpage.
Examples
Example − Display a Counter
In this app we will display a counter that initially starts at 0 and updates to 1 after 1 second as we will simulate it by 1000 milliseconds. So the code for this app is as follows −
// Importing React and ReactDOM import React from 'react'; import ReactDOM from 'react-dom'; // Creating a React element for a counter const counterElement = <p>Count: 0</p>; // Render the counter element into a detached DOM node const domContainer = document.createElement('div'); ReactDOM.createRoot(domContainer).render(counterElement); document.body.appendChild(domContainer); // Update after 1 second setTimeout(() => { const updatedCounterElement = <p>Count: 1</p>; ReactDOM.createRoot(domContainer).render(updatedCounterElement); }, 1000);
Output

Example − Create a simple Form
In this app we will create a simple form with a text input and a submit button in React JS. So the code for the same is as follows −
// Importing React and ReactDOM import React from 'react'; import ReactDOM from 'react-dom'; // Create a React element for a simple form const formElement = ( <form> <label> Name: <input type="text" /> </label> <br /> <button type="submit">Submit</button> </form> ); // Render the form element into a detached DOM node const domContainer = document.createElement('div'); ReactDOM.createRoot(domContainer).render(formElement); document.body.appendChild(domContainer);
Output

Example − Display a List with Dynamic Content
So now we will create an app in which we will display a dynamic list of items (initially fruits) and update the list to include different fruits after 2 seconds. So the code for this app is as follows −
// Importing React and ReactDOM import React from 'react'; import ReactDOM from 'react-dom'; // Create a React element for a dynamic list const items = ['Apple', 'Banana', 'Orange']; const listElement = ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); // Render the list element into a detached DOM node const domContainer = document.createElement('div'); ReactDOM.createRoot(domContainer).render(listElement); document.body.appendChild(domContainer); // Update after 2 seconds setTimeout(() => { const updatedItems = ['Grapes', 'Kiwi', 'Mango']; const updatedListElement = ( <ul> {updatedItems.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); ReactDOM.createRoot(domContainer).render(updatedListElement); }, 2000);
Output

Note
It is important to note that for renderIntoDocument() to work correctly, some global objects like window, window.document, and window.document.createElement should be available. This makes sure that React can access the DOM and perform rendering operations.
Summary
So we have seen how renderIntoDocument() method works and how we can put this function into practice. This requires a Document Object Model (DOM) to function correctly. It is similar to a specific set of operations where a DOM container is created, and the provided React element is rendered into it using ReactDOM.createRoot().render().
To Continue Learning Please Login