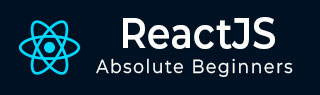
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - isCompositeComponent()
React JS is all about dividing our app into smaller components, and each of these components has its own lifecycle. React provides built-in methods that we can use at different stages in a component’s life.
In React JS, the isCompositeComponent() method is basically used to check if an instance is a user-defined component. It helps us find whether a particular element is a custom component created by the developer or not. So we can say that it helps us find out if a particular part of our app is a component we have created or not.
Syntax
isCompositeComponent(instance)
Parameters
instance − This is the element that we want to check. This method is used to check that an element or component instance is a user-defined component or not. So we can check any React element or component instance to see if it is a custom component or built-in.
Return Value
It returns a boolean value, true if the provided element is a user-defined component, false otherwise.
Examples
Example 1
Now, we will create a simple React app that shows how we can use this method. We will create a basic component called App and use isCompositeComponent() in it.
import React from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; // Define App Component const App = () => { // Function to show isCompositeComponent() function myFunction() { var a = isCompositeComponent(el); console.log("It is an element :", a); } const el = <div> <h1>element</h1> </div> // Return our JSX code return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={myFunction}>Click me !!</button> </div> ); } export default App;
Output

In the code, we saw a simple component called ‘App.’ There is a function in this component named ‘myFunction’ that uses isCompositeComponent() to figure out that the ‘el’ element is a user-defined component. When we click the ‘Click me!!’ button, the outcome is logged in the console.
Example 2
This app consists of a simple functional component named FunctionalComponent. The main App component renders a button that, when clicked, checks whether FunctionalComponent is a composite component using the isCompositeComponent() method.
import React from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; const FunctionalComponent = () => { return <div>Hello from FunctionalComponent!</div>; }; const App = () => { function checkComponent() { const isComposite = isCompositeComponent(<FunctionalComponent />); console.log("Is FunctionalComponent a composite component?", isComposite); } return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={checkComponent}>Check FunctionalComponent</button> </div> ); }; export default App;
Output

Example 3
This app features a simple class component named ClassComponent. The main App component renders a button that, when clicked, checks whether ClassComponent is a composite component using the isCompositeComponent() method.
import React, { Component } from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; class ClassComponent extends Component { render() { return <div>Hello from ClassComponent!</div>; } } const App = () => { function checkComponent() { const isComposite = isCompositeComponent(<ClassComponent />); console.log("Is ClassComponent a composite component?", isComposite); } return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={checkComponent}>Check ClassComponent</button> </div> ); }; export default App;
Output

Summary
The isCompositeComponent() method is useful for figuring out whether a specific element of our React app is a component we created. For user-defined elements, it returns true, otherwise, it returns false.
To Continue Learning Please Login