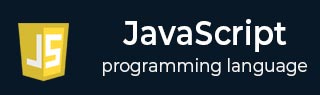
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Number toString() Method
The JavaScript Number toString() method is used to change the variable type to string and returns a string representation. It accepts an optional parameter called 'radix', which is an integer that represents the base in the mathematical numeral system. The range of this parameter is between '2' and '36, and the default value of this parameter is '10'.
This method throws a 'RangeError' exception if the radix parameter value is not in the range of [2, 36].
Note: Both '0' and '-0' are represented as "0", while Infinity is represented as "Infinity" and NaN as "NaN".
Syntax
Following is the syntax of JavaScript Number toString() method −
toString(radix)
Parameters
This method accepts an optional parameter called 'radix', which is described below −
- radix (optional) − Specifying the base to use for representing the number value.
Return value
This method returns a string representation of the specified number value.
Example 1
The example below will demonstrate how to use the JavaScript Number toString() method.
<html> <head> <title>JavaScript toString() Method</title> </head> <body> <script> const val1 = 5494; const val2 = "1234"; document.write("Given values = ", val1 , " and ", val2); document.write("<br>Result 1 = ", val1.toString()); document.write("<br>Result 2 = ", val2.toString()); </script> </body> </html>
Output
Following is the output of the above program −
Given values = 5494 and 1234 Result 1 = 5494 Result 2 = 1234
Example 2
If the radix parameter is set to 20, this method returns a string representing of the specified number value using base 20.
<html> <head> <title>JavaScript toString() Method</title> </head> <body> <script> const val = 1234; const radix = 20; document.write("Given value = ", val); document.write("<br>Radix value = ", radix); document.write("<br>Result = ", val.toString(radix)); </script> </body> </html>
Output
After executing the above program, it will return a string representation of the number 1234 as −
Given value = 1234 Radix value = 20 Result = 31e
Example 3
Let's take a look at an example of the toString() method in real-time usage. In the following example, we used the toString() method inside the custom function called hexColor(). We use the Math.abs().toString() function to return a string representation of the given or passed value.
<html> <head> <title>JavaScript toString() Method</title> </head> <body> <script> function hexColor(col){ if(col < 256){ return Math.abs(col).toString(16); } else{ return 0; } } const val = 230; const val2 = "40"; document.write("Given values = ", val, " and ", val2); document.write("<br>Result 1 = ", hexColor(val)); document.write("<br>Result 2 = ", hexColor(val2)); </script> </body> </html>
Output
Once the above program is executed, it return a string representation for number 230 and 40 as −
Given values = 230 and 40 Result 1 = e6 Result 2 = 28
Example 4
If the value of the optional parameter 'radix' is not within the range of [2, 100], the toString() method will throw a 'RangeError' exception.
<html> <head> <title>JavaScript toString() Method</title> </head> <body> <script> const val = 1234; const radix = 1; document.write("Given value = ", val); document.write("<br>Radix value = ", radix); try { document.write("<br>Result = ", val.toString(radix)); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
The above program throws a 'RangeError' exception −
Given value = 1234 Radix value = 1 RangeError: toString() radix argument must be between 2 and 36
To Continue Learning Please Login