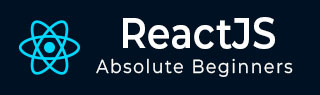
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.toJSON() Method
For React components, the TestRenderer package is really useful. It can show how components appear in JavaScript without using a web page or mobile setup.
For example, photographing the look of React components, like a tree structure. This can be done without the usage of a web browser or jsdom. It is useful for testing the look of items without needing to use the actual web environment.
testRenderer.toJSON() is a method in React's testing library that returns an object. This tree only includes platform-specific nodes and their properties, like <div> or <View>. It has no user-written components. This method is mainly used in snapshot testing, in which a "snapshot" of the rendered component is captured and compared to a previously stored snapshot to ensure that our UI does not change unintentionally.
Syntax
testRenderer.toJSON()
Parameters
There are no parameters taken by the method. We use it on a TestRenderer instance directly.
Return Value
The method returns an object that represents the displayed tree. This tree contains platform-specific nodes like <div> and <View>, as well as related properties. It does not, however, include any user-written components.
Here's a simple usage example
import React from 'react'; import TestRenderer from 'react-test-renderer'; const MyComponent = () => <div>Hello, World!</div>; const testRenderer = TestRenderer.create(<MyComponent />); const tree = testRenderer.toJSON(); console.log(tree);
In this example, a tree would be an object representing the rendered structure of the MyComponent.
Examples
Example − Displaying a Simple Message
This app is a simple React component that renders a simple message inside a <div>. The message is "Hello, Simple React App 1!". It is a simple example to show the basic usage of testRenderer.toJSON(). When we run this app, it will log an object showing the rendered tree, containing a <div> node with the message. So the code for this app is as follows −
import React from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { return <div>Hello, Simple React App 1!</div>; }; export default App; const testRenderer = TestRenderer.create(<App />); const tree = testRenderer.toJSON(); console.log(tree);
Output

Example − Creating a List App
In this app, we will create a React component that creates a list (<ul>) with three items (<li>). The items are "Item 1", "Item 2", and "Item 3". The list is dynamically generated with the help of the map function. When we run this app, it will log an object representing the rendered tree, capturing the structure of the list and its items.
import React from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { const items = ['Item 1', 'Item 2', 'Item 3']; return ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); }; export default App; const testRenderer = TestRenderer.create(<App />); const tree = testRenderer.toJSON(); console.log(tree);
Output

Example − Interactive Button App
This app creates a React component with a state variable (count) and a button. The state is initially set to 0, and it is displayed in a paragraph (<p>) element. Clicking the button increments the count. This example shows a more interactive component using state management. When we run this app, it will log an object representing the rendered tree, including the structure of the paragraph and button elements.
import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; import './App.css'; const App = () => { const [count, setCount] = useState(0); return ( <div className='App'> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default App; const testRenderer = TestRenderer.create(<App />); const tree = testRenderer.toJSON(); console.log(tree);
Output

Summary
The testRenderer.toJSON() is a method in React's testing library which provides a representation of the rendered component tree. So we have seen how it works and three different apps using it. These three apps provide simple examples of how to use testRenderer.toJSON() in different situations, from basic rendering to more interactive components. The console objects represent the structure of the rendered components, which can be useful for testing and troubleshooting.
To Continue Learning Please Login