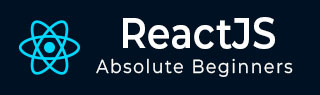
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.findAll() Method
The function testInstance.findAll(test) is a synthetic or general image of a method that could exist in a programming context. The testInstance represents an instance or object in a program. The term 'testInstance' is simply a placeholder and should be changed with the real name of the instance with which we are working. The findAll method or function can be called on the testInstance object. It means that we want to locate or collect something based on a specific condition.
Syntax
testInstance.findAll(test)
Parameters
test − As an argument, we pass another function called test. This is a function defined somewhere else in our code. This function checks whether a specific condition is met for each item in the collection.
Return Value
The findAll method returns a collection, like an array, list, or another data structure, containing all the items from testInstance that meet the conditions defined in the test function.
Examples
Example − Filtering Todo List
First we will create an app in which we will have a TodoList component. This component provides a simple todo list with the ability to filter and display completed or non-completed todos based on user input. So the code for this app is as follows −
TodoList.js
import React, { useState } from 'react'; const TodoList = ({ todos }) => { const [showCompleted, setShowCompleted] = useState(false); // findAll method const findAll = (testFunction) => (todos || []).filter(testFunction); const test = (todo) => { return showCompleted ? todo.completed : !todo.completed; }; const filteredTodos = findAll(test); return ( <div> <label> <input type="checkbox" checked={showCompleted} onChange={() => setShowCompleted(!showCompleted)} /> Show Completed </label> <ul> {filteredTodos.map((todo) => ( <li key={todo.id}>{todo.text}</li> ))} </ul> </div> ); }; export default TodoList;
Output

The component shows a checkbox that allows us to choose between showing completed and non completed tasks. The filtered todos are then displayed in an unordered list.
Example − Filtering Product App
In this example we will create a ProductList component. This component provides a simple product list with the ability to filter and display products as per the selected category. Users can choose a category from the dropdown, and the list updates accordingly.
ProductList.js
import React, { useState } from 'react'; const ProductList = ({ products }) => { const [selectedCategory, setSelectedCategory] = useState('all'); // findAll method const findAll = (testFunction) => (products || []).filter(testFunction); const test = (product) => { return selectedCategory === 'all' || product.category === selectedCategory; }; const filteredProducts = findAll(test); return ( <div> <label> Select Category: <select value={selectedCategory} onChange={(e) => setSelectedCategory(e.target.value)} > <option value="all">All</option> <option value="electronics">Electronics</option> <option value="clothing">Clothing</option> </select> </label> <ul> {filteredProducts.map((product) => ( <li key={product.id}>{product.name}</li> ))} </ul> </div> ); }; export default ProductList;
Output

Example − Filtering Users List
In this app, UserList is a functional component that accepts a prop called users, and an array of user items.The findAll method filters users as per the provided testFunction. It checks if the user is undefined or null and uses an empty array in that cases. The test function is used as the filtering criteria. It checks whether to show active users (showActive === true) or inactive users.
UserList.js
import React, { useState } from 'react'; const UserList = ({ users }) => { const [showActive, setShowActive] = useState(true); // findAll method const findAll = (testFunction) => (users || []).filter(testFunction); const test = (user) => { return showActive ? user.status === 'active' : user.status === 'inactive'; }; const filteredUsers = findAll(test); return ( <div> <label> <input type="checkbox" checked={showActive} onChange={() => setShowActive(!showActive)} /> Show Active Users </label> <ul> {filteredUsers.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> </div> ); }; export default UserList;
Output

Overall, this component provides a simple user list that can be filtered and shown based on active/inactive status. Users can toggle the checkbox to display active or inactive users.
Summary
The findAll method provides a convenient way to filter and collect items from a larger set based on specific criteria defined in the test function. The actual result is a new collection containing only the items that pass the test. So we have seen different apps using this function to get the practical exposure of this method in testing.
To Continue Learning Please Login