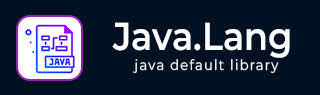
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - toString() Method
Description
The method is used to get a String object representing the value of the Number Object.
If the method takes a primitive data type as an argument, then the String object representing the primitive data type value is returned.
If the method takes two arguments, then a String representation of the first argument in the radix specified by the second argument will be returned.
Syntax
Following are all the variants of this method −
String toString() static String toString(int i)
Parameters
Here is the detail of parameters −
i − An int for which string representation would be returned.
Return Value
toString() − This returns a String object representing the value of this Integer.
toString(int i) − This returns a String object representing the specified integer.
Getting String Representation of an Integer and int Value Example
In this example, we're showing the usage of toString() method to get String representation of a int or Integer object. We've created a Integer variable x and initialized it with a value. Then using toString() and toString(int), we've printed the string representation of first Integer object and then of an int.
package com.tutorialspoint; public class Test { public static void main(String args[]) { Integer x = 5; System.out.println(x.toString()); System.out.println(Integer.toString(12)); } }
Output
This will produce the following result −
5 12
Getting String Representation of a Double and double Value Example
In this example, we're showing the usage of toString() method to get String representation of a double or Double object. We've created a Double variable x and initialized it with a value. Then using toString() and toString(double), we've printed the string representation of first Double object and then of an double.
package com.tutorialspoint; public class Test { public static void main(String args[]) { Double x = 5.0; System.out.println(x.toString()); System.out.println(Double.toString(12.0)); } }
Output
This will produce the following result −
5.0 12.0
Getting String Representation of a Long and long Value Example
In this example, we're showing the usage of toString() method to get String representation of a long or Long object. We've created a Long variable x and initialized it with a value. Then using toString() and toString(long), we've printed the string representation of first Long object and then of an long.
package com.tutorialspoint; public class Test { public static void main(String args[]) { Long x = 5L; System.out.println(x.toString()); System.out.println(Long.toString(12L)); } }
Output
This will produce the following result −
5 12
To Continue Learning Please Login