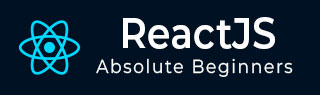
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - TestRenderer.act() Method
React testing is important for making sure our components function as expected. So we will look at the TestRenderer.act() function, which is a useful tool for making testing easier.
TestRenderer.act() is a function available in the 'react-test-renderer' library. This method allows us to prepare a React component for assertions. It is similar to the act() helper from 'react-dom/test-utils,' and it makes it easy to test TestRenderer components.
Syntax
TestRenderer.act(callback);
To get started, we will have to import the necessary functions −
import { create, act } from 'react-test-renderer';
Let us consider an example in which we have a component called App that gets a prop called value −
import App from './app.js'; // render the component let root; act(() => { root = create(<App value={1} />); }); // make assertions on root expect(root.toJSON()).toMatchSnapshot();
In this code, we create an instance of the App component with a prop of value equal to 1. The act() method wraps this creation process, to make sure that the component is ready for testing.
Now, we will update the component with different props −
// update with some different props act(() => { root.update(<App value={2} />); }); // make assertions on root expect(root.toJSON()).toMatchSnapshot();
With the usage of act() again, we safely update the component with new props and make assertions on the updated component.
Examples
Let's put this information to use by creating small React apps with the TestRenderer.act(callback) function. We will show how this function can be useful in a number of testing scenarios.
Example − Basic Component
In this first app, we will check a simple thing - a basic part of a React program we call "BasicComponent." It is like a small piece of our project. This part does not need any extra information. We want to see if it looks right when we show it on the screen. The way we check is by creating it and making sure it looks just like we expected.
BasicComponent.js
import React from 'react'; const BasicComponent = () => { return ( <div> <h1>Hello, I'm a Basic Component!</h1> <p>This is a simple React component for testing.</p> </div> ); }; export default BasicComponent;
App.js
import { create, act } from 'react-test-renderer'; // Import the component import BasicComponent from './BasicComponent.js'; let root; act(() => { root = create(<BasicComponent />); }); expect(root.toJSON()).toMatchSnapshot();
Output

In this example, BasicComponent is a basic React functional component that creates a div with a h1 heading and a p paragraph. This component is meant for testing, and its output will be compared to the snapshot in our test code.
Example − Component with Props
The second app is for testing a React component called ComponentWithProps, which gets a name prop with the value "John." This example shows how to use TestRenderer.act() to create an instance of a component with particular features and confirm that the rendered result matches the desired snapshot.
ComponentWithProps.js
import React from 'react'; const ComponentWithProps = (props) => { return ( <div> <h1>Hello, {props.name}!</h1> <p>This component receives a prop named "name" and displays a greeting.</p> </div> ); }; export default ComponentWithProps;
App.js
import { create, act } from 'react-test-renderer'; // Import the component import ComponentWithProps from './ComponentWithProps.js'; let root; act(() => { root = create(<ComponentWithProps name="John" />); }); expect(root.toJSON()).toMatchSnapshot();
Output

In the code above ComponentWithProps is another simple functional component that receives a prop named name. This prop is used to create a greeting that shows in the h1 heading. The paragraph also gives a brief explanation for the component.
Example − Dynamic Component
In this program we will show how to test a dynamic React component called DynamicComponent. This component's lifecycle is going to include changes like state updates or prop changes. The test uses setting up the component and recreating dynamic changes by updating it. This shows how TestRenderer.act() can deal with dynamic component behavior.
DynamicComponent.js
import React, { useState, useEffect } from 'react'; const DynamicComponent = () => { const [count, setCount] = useState(0); useEffect(() => { // Dynamic changes after component mount const interval = setInterval(() => { setCount((prevCount) => prevCount + 1); }, 1000); // Clean up interval on component unmount return () => clearInterval(interval); }, []); return ( <div> <h1>Dynamic Component</h1> <p>This component changes dynamically. Count: {count}</p> </div> ); }; export default DynamicComponent;
App.js
import { create, act } from 'react-test-renderer'; // Import the component import DynamicComponent from './DynamicComponent.js'; let root; act(() => { root = create(<DynamicComponent />); }); // dynamic changes act(() => { root.update(<DynamicComponent />); }); expect(root.toJSON()).toMatchSnapshot();
Output

In this example, DynamicComponent is a functional component that makes use of the useState and useEffect hooks. It starts a timer using setInterval to increment the count every second after initializing a count state. This represents the component's dynamic changes over time.
Also the above examples show how TestRenderer.act() can be used to test various types of React components. By following these patterns, we can simplify our testing process and ensure the reliability of our React applications.
Note
It is important to use TestRenderer.act() when working with the react-test-renderer to ensure compatibility with React's internal behavior and to correct and reliable test results.
Summary
TestRenderer.act() is a function available in the react-test-renderer library. Its main purpose is to help with testing React components. It ensures that our tests are performed in a way that closely resembles how React updates the user interface. So we have seen how it works and how we can use this function in different scenarios.
To Continue Learning Please Login