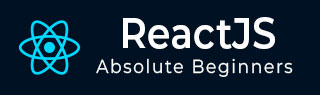
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.type Property
The React JS library is all about dividing the program into many components. Each component has a unique lifecycle. React provides some built-in methods that we can override at various points of the component's life-cycle.
So in this tutorial we will learn how to use the testInstance.type property. This property is used to get the component type which is related to the test instance.
Syntax
testInstance.type
Let us understand this property by an example. When a developer uses testInstance.type in their code, they are asking the computer to tell them what kind of part they are working with. This is important in making sure the software works easily and performs as expected.
For example, an item of code like button.type shows that the developer wants to know the kind of a button in their programs. The computer will then answer with something like "Button," helping the developer in better understanding and managing their code.
Return Value
The testInstance.type returns the type or kind of a computer program component. In simple terms, it provides the developer with information about an element of the application.
Examples
Example − DivTypeApp
In this app we will use TestRenderer to create a simple React component (a <div> element) and log its type to the console. The JSX return is a basic paragraph element displaying an example of the testRenderer.type property. See the code for this app below −
import React from 'react'; import TestRenderer from 'react-test-renderer'; // Defining our DivTypeApp Component const DivTypeApp = () => { // Function to demonstrate TestRenderer.type Property function testFunction() { const renderer = TestRenderer.create( <div>The testRenderer.type Property</div> ); const mytype = renderer.root; console.log(mytype.type); } testFunction(); // Returning our JSX code return <> <p> The testRenderer.type Property Example </p> </>; } export default DivTypeApp;
Output

Example − ButtonTypeApp
In this app we will use TestRenderer to create a React component (a button element) and then log its type to the console. The JSX return is a simple paragraph element displaying an example of the testRenderer.type property. So the code for the same is given below −
import React from 'react'; import TestRenderer from 'react-test-renderer'; const ButtonTypeApp = () => { function testButtonType() { const renderer = TestRenderer.create( <button>Click me</button> ); const buttonType = renderer.root; console.log(buttonType.type); } testButtonType(); return ( <> <p>ButtonTypeApp: Testing testInstance.type with a button.</p> </> ); }; export default ButtonTypeApp;
Output

Example − HeadingTypeApp
In this app we will use TestRenderer to create a React component (an <h1> heading), log its type to the console (which is "h1" in this case), and return a JSX structure with an explanatory paragraph. This is a basic example of testing the testInstance.type property with a heading element. Here is the code for this app −
import React from 'react'; import TestRenderer from 'react-test-renderer'; const HeadingTypeApp = () => { function testHeadingType() { const renderer = TestRenderer.create( <h1>Hello, TestInstance!</h1> ); const headingType = renderer.root; console.log(headingType.type); // Output: "h1" } testHeadingType(); return ( <> <p>HeadingTypeApp: Testing testInstance.type with a heading.</p> </> ); }; export default HeadingTypeApp;
Output

Summary
The testInstance.type is similar to placing name tags on different puzzle pieces in a computer program. It helps developers in knowing and organizing their code, making it easier for the computer to understand what each component is supposed to perform. AS we have created three different examples using testInstance.type property. By practicing these examples we can understand its practical use.
To Continue Learning Please Login