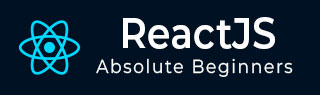
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.instance Property
The component instance is important for class components because it helps manage the internal state and behavior of that specific component. When we reference this inside a class component, we are referring to its instance. This allows us to interact with the state of the component, lifecycle methods, and other functionalities.
In React testing, there is a concept of testInstance.instance. This property provides access to the component instance related to a specific test instance. It allows us to inspect and interact with the component's internal workings during testing.
Syntax
testInstance.instance
Parameters
testInstance − Represents a rendered React component in the testing environment.
.instance− A property or method that allows us to access the actual instance of the React component being tested.
The component instance associated with this test instance. Because function components do not have instances, it is only possible for class components. It is equivalent to this value within the given component.
Return Value
testInstance.instance returns the component instance related with a specific test instance. It gives access to the underlying instance of a React component, particularly in the terms of testing.
Examples
Example − Testing Component State
In this example we will create a Counter app in which we will have a simple React class component that manages a counter state. It will have a method to increment the counter value. And also we will create a test file for this component. The test will make sure that the component correctly increments the counter when the increment method is called. And for this we will use testInstance.instance to access the component instance and trigger the increment method. So the code for both Counter component and its test file are as follows −
Counter.js
import React, { Component } from 'react'; class Counter extends Component { constructor() { super(); this.state = { count: 0 }; } increment() { this.setState((prevState) => ({ count: prevState.count + 1 })); } render() { return ( <div> <p>Count: {this.state.count}</p> </div> ); } } export default Counter;
Counter.test.js
import { render, screen } from '@testing-library/react'; import Counter from './Counter'; test('increments the count', () => { const { testInstance } = render(<Counter />); // Trigger the increment method testInstance.instance.increment(); // Check if the count has been updated expect(screen.getByText(/Count:/).textContent).toBe('Count: 1'); });
Output

Example − Testing Component Lifecycle
Now we will create a LifecycleExample app in which we will have a React class component that displays a message after it has mounted. It will utilizes the componentDidMount lifecycle method to update the state after mounting. And secondly we will also create its test files to check the component's lifecycle by accessing the component instance using testInstance.instance. It will verify that the state is updated as expected after the component has mounted and checks if the rendered output matches the updated state. So the code is given below for this −
LifecycleExample.js
import React, { Component } from 'react'; class LifecycleExample extends Component { constructor() { super(); this.state = { message: '' }; } componentDidMount() { this.setState({ message: 'Component has mounted!' }); } render() { return <p>{this.state.message}</p>; } } export default LifecycleExample;
LifecycleExample.test.js
import { render, screen } from '@testing-library/react'; import LifecycleExample from './LifecycleExample'; test('checks component lifecycle', () => { const { testInstance } = render(<LifecycleExample />); // Check the state after mounting expect(testInstance.instance.state.message).toBe('Component has mounted!'); // Check if the rendered output matches the updated state expect(screen.getByText(/Component has mounted!/).textContent).toBe('Component has mounted!'); });
Output

Example − Interaction with Component Methods
In the third example we will have a ClickButton app which is a React class component to represent a button that toggles its state when it is clicked. It will also have a method, handleClick, which will update the state to show that the button has been clicked.
And next we will create its corresponding test file to interact with the component by clicking the button using the fireEvent.click method. It will then use testInstance.instance to check if the component state has been updated as expected.
ClickButton.js
import React, { Component } from 'react'; class ClickButton extends Component { constructor() { super(); this.state = { clicked: false }; } handleClick() { this.setState({ clicked: true }); } render() { return ( <button onClick={() => this.handleClick()}> {this.state.clicked ? 'Clicked!' : 'Click me'} </button> ); } } export default ClickButton;
ClickButton.test.js
import { render, fireEvent, screen } from '@testing-library/react'; import ClickButton from './ClickButton'; test('handles button click', () => { const { testInstance } = render(<ClickButton />); // Trigger the handleClick method fireEvent.click(screen.getByText('Click me')); // Check if the component state has been updated expect(testInstance.instance.state.clicked).toBe(true); expect(screen.getByText(/Clicked!/).textContent).toBe('Clicked!'); });
Output

Summary
Understanding component instances in React is important for effective component management and testing, especially in the context of class components. By knowing this concept, we will be able to manage the complexities of the React apps. We have created three apps using the testInstance.instance method. These apps and testing scenarios show various elements of testing React components, like state management, lifecycle methods, and interaction with component methods. The use of testInstance.instance allows complete testing and component internal modifications during testing.
To Continue Learning Please Login