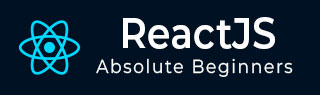
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
There is a useful tool in React called findAllInRenderedTree. This tool helps us in locating specific parts, known as components, within our app.
Consider our React app to be a large tree, with each component acting as a branch. The findAllInRenderedTree method acts as a friendly explorer, traversing each branch and determining whether or not it matches a test.
This is how it works
Input − The explorer receives two items: the tree (our React app) and a test (a rule that will help us find what we are looking for).
Traversal − The explorer walks through each component of the tree one by one.
Testing − The explorer uses testing for each component. If the component passes the test (the test returns true), the explorer adds it to a list.
Results − Finally, we will be given a list of all the components that passed the test.
Syntax
findAllInRenderedTree( tree, test )
Parameters
tree (React.Component) − The tree to traverse's root component.
test (function) − A test function that takes an input and returns a boolean value. This function determines if a component should be included in the outcome.,
Return Value
This function returns an array of components that passed the test.
Examples
Example 1
To find all completed items in a to-do list, use findAllInRenderedTree.
Create a React app that includes a to-do list. To mark a task as completed, add a checkbox next to it. We will use findAllInRenderedTree to identify and display all completed jobs separately.
import React, { useState } from 'react'; import './App.css'; const TodoApp = () => { const [tasks, setTasks] = useState([ { id: 1, text: 'Complete task 1', completed: false }, { id: 2, text: 'Finish task 2', completed: true }, // Add more tasks if needed ]); const findCompletedTasks = () => { // Using findAllInRenderedTree to find completed tasks const completedTasks = findAllInRenderedTree(tasks, (task) => task.completed); console.log('Completed Tasks:', completedTasks); }; return ( <div className='App'> <h2>Todo List</h2> {tasks.map((task) => ( <div key={task.id}> <input type="checkbox" checked={task.completed} readOnly /> <span>{task.text}</span> </div> ))} <button onClick={findCompletedTasks}>Find Completed Tasks</button> </div> ); }; export default TodoApp;
Output

Example 2
In this app we have to identify and display all components related to colors in a palette, so we will use findAllInRenderedTree.
Create a React app using a color palette, with each color acting as a component. To discover and display all color components, use findAllInRenderedTree.
import React from 'react'; import './App.css'; const ColorPaletteApp = () => { const colors = ['red', 'blue', 'green', 'yellow', 'purple']; const findColorComponents = () => { // Using findAllInRenderedTree to find color components const colorComponents = findAllInRenderedTree(colors, (color) => color); console.log('Color Components:', colorComponents); }; return ( <div className='App'> <h2>Color Palette</h2> {colors.map((color) => ( <div key={color} style={{ backgroundColor: color, height: '50px', width: '50px' }}></div> ))} <button onClick={findColorComponents}>Find Color Components</button> </div> ); }; export default ColorPaletteApp;
Output

Example 3
In this app we will locate and evaluate all response components in an interactive quiz. So create a React app that includes an interactive quiz with questions and multiple-choice responses. Find and assess the selected answers using findAllInRenderedTree, offering feedback depending on correctness.
import React, { useState } from 'react'; import './App.css'; const QuizApp = () => { const [questions, setQuestions] = useState([ { id: 1, text: 'What is the capital of France?', options: ['Berlin', 'London', 'Paris'], correctAnswer: 'Paris' }, { id: 2, text: 'Which planet is known as the Red Planet?', options: ['Earth', 'Mars', 'Venus'], correctAnswer: 'Mars' }, ]); const checkAnswers = () => { // Using findAllInRenderedTree to find selected answers const selectedAnswers = findAllInRenderedTree(questions, (question) => question.selectedAnswer); console.log('Selected Answers:', selectedAnswers); }; const handleOptionClick = (questionId, selectedOption) => { setQuestions((prevQuestions) => prevQuestions.map((question) => question.id === questionId ? { ...question, selectedAnswer: selectedOption } : question ) ); }; return ( <div className='App'> <h2>Interactive Quiz</h2> {questions.map((question) => ( <div key={question.id}> <p>{question.text}</p> {question.options.map((option) => ( <label key={option}> <input type="radio" name={`question-${question.id}`} value={option} onChange={() => handleOptionClick(question.id, option)} /> {option} </label> ))} </div> ))} <button onClick={checkAnswers}>Check Answers</button> </div> ); }; export default QuizApp;
Output

Summary
These examples show how the findAllInRenderedTree method can be used in a variety of situations, from task management to browsing color palettes and generating interactive quizzes. Each app makes use of the method to locate and interact with certain components in the React tree.
To Continue Learning Please Login