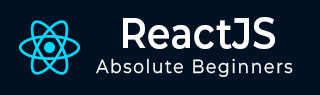
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.unmount() Method
Unmounting an in-memory tree can sound difficult, but it is a simple operation which requires executing the correct lifecycle events. We will talk about the stages of using the testRenderer.unmount() method in this tutorial.
An in-memory tree is a representation of our React components that exist in computer memory. It is similar to a virtual version of our user interface.
We have to uninstall or unmount a component from the in-memory tree at times. This can be due to the fact that we no longer want it or that we are cleaning up after a test.
The testRenderer.unmount() method in React testing is used to unmount() method a component. Imagine it like deleting a virtual sign or display.
Syntax
testRenderer.unmount()
Parameters
The unmount() method does not take any parameters.
Return Value
The unmount() method generates no output. When we call unmount(), React performs its work without returning anything.
Examples
Unmounting an in-memory tree is a simple process using testRenderer.unmount(). So we will see the usage of this function with different examples −
Example − Basic Component unmount
// Basic Component Unmount import React from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { // Render a simple component const testRenderer = TestRenderer.create(<div>Hello, App!</div>); //unmounting the component testRenderer.unmount(); // The component is now removed from the tree return null; // This component doesn't render anything visible } export default App;
Output

As the component is unmounted immediately, there will not be any visible output. The component is removed from the in-memory tree right after rendering.
Example − Conditional Rendering and Unmount
In this app we will render a component conditionally based on the state (showComponent). After a delay of 2 seconds, the state will be updated to hide the component, and the testRenderer.unmount() function is used to show unmounting.
// Conditional Rendering and Unmount import React, { useState, useEffect } from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { const [showComponent, setShowComponent] = useState(true); useEffect(() => { // After a delay, toggle the state to hide the component const timeout = setTimeout(() => { setShowComponent(false); }, 2000); return () => clearTimeout(timeout); // Cleanup to avoid memory leaks }, []); return ( <div> {showComponent && <div>Hello, App!</div>} {!showComponent && ( // unmounting the component after it is hidden <TestRenderer.unmount /> )} </div> ); } export default App;
Output

Initially, we will see "Hello, App!" displayed. After 2 seconds, the component disappears, and the testRenderer.unmount() function is invoked. The output will be an empty page.
Example − Dynamic Component Unmount with State
This app will dynamically render or unmount a component based on a button click. The component visibility will be controlled by the componentVisible state, and the testRenderer.unmount() function will be used when the component is hidden.
// Dynamic Component Unmount with State import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { const [componentVisible, setComponentVisible] = useState(true); const handleUnmount = () => { // Toggle the state to hide or show the component setComponentVisible(!componentVisible); }; return ( <div> {componentVisible && <div>Hello, App!</div>} <button onClick={handleUnmount}> {componentVisible ? 'Unmount Component' : 'Mount Component'} </button> {!componentVisible && ( // unmounting the component based on state <TestRenderer.unmount /> )} </div> ); } export default App;
Output

Initially, we will see "Hello, App!" and a button. Clicking the button changes the visibility of the component, and when it is hidden, the testRenderer.unmount() function is invoked.
Summary
The testRenderer.unmount() is a useful tool for cleaning up components in our React tests. Remember, it is a one-liner with no parameters, no return values, just a simple command to tidy up our in-memory tree. We have created three different examples to show various scenarios in which the testRenderer.unmount() function is used.
To Continue Learning Please Login