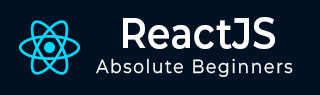
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testRenderer.getInstance() Method
The concept we will talk about in this tutorial is known as testRenderer.getInstance().
testRenderer.getInstance() functions as a debugging tool for developers. Suppose we are creating a website or an app and want to learn more about the key component of our creation - the root element. This tool will assist us in doing this task.
In simple words, the testRenderer.getInstance() function is a tool that helps developers understand and inspect the main component of what they are creating, but it has limits when dealing with objects formed by function components.
Syntax
testRenderer.getInstance()
Parameters
No parameters needed for this method. When we use this method, we do not need to give it any special instructions or information.
Return Value
The testRenderer.getInstance() method returns the root element’s instance. In simple terms, it provides an in-depth understanding or snapshot of the key elements of what developers are creating.
Examples
Example
In this example, we will have a simple app that shows a list of characters. To get information about the root element, which is the CharacterList component, we will use testRenderer.getInstance(). And we will use a simplified render function for illustration purposes.
import React from 'react'; import ReactDOM from 'react-dom'; // React component function CharacterList({ characters }) { return ( <div> <h1>List of Characters</h1> <ul> {characters && characters.map((character, index) => ( <li key={index}>{character}</li> ))} </ul> </div> ); } export default CharacterList; // Function to render a React component function render(element) { const container = document.createElement('div'); ReactDOM.render(element, container); return { getInstance: () => container.firstChild, }; } // list of characters const characters = ['Ankit', 'Babita', 'Chetan']; // Render the characters using React const testRenderer = render(<CharacterList characters={characters} />); // Use testRenderer.getInstance() to get details const characterListInstance = testRenderer.getInstance(); // Display information about the root element console.log(`Details about CharacterList: `, characterListInstance);
Output

So after running the app we can see on the console the List of Characters and inside the list we can see three elements.
Example − Counter App
In this app, the Counter component renders a counter with increment and decrement buttons. We will use testRenderer.getInstance() to interact with the rendered component, simulating a click on the Increment button. So the code for this app is as follows −
import React, { useState } from 'react'; import ReactDOM from 'react-dom'; // Counter component function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; return ( <div> <h1>Counter App</h1> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); } export default Counter; // Function to render a React component function render(element) { const container = document.createElement('div'); ReactDOM.render(element, container); return { getInstance: () => container.firstChild, }; } // Render the Counter component using React const testRenderer = render(<Counter />); // interacting with the counter testRenderer.getInstance().querySelector('button').click(); // Use testRenderer.getInstance() const counterInstance = testRenderer.getInstance(); // Display information about the root element console.log(`Details about Counter: `, counterInstance);
Output

Summary
As a result, testRenderer.getInstance() is a developer tool that allows them to explore the main component of their project in more detail. It functions similarly to a magnifying glass, although it cannot collect characters from functional components. Understanding this concept helps developers to create more effective and efficient websites and apps.
To Continue Learning Please Login