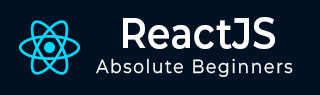
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - TestRenderer.create() Method
The TestRenderer package is very useful for React components. It can show how components appear in JavaScript without the requirement for a web page or mobile setup.
For example photographing the look of React components, like a tree structure. This package performs this without the use of a web browser or jsdom. It is helpful for checking how things look without having to use the actual web environment.
The TestRenderer.create() function is a React method to make testing easier. It allows us to create a particular kind of instance to test how React components behave without relying on an actual web browser. So basically this function acts as an interface, creating a TestRenderer object for us.
Syntax
TestRenderer.create(element, options);
Parameters
element − This is the React component to be tested. We give it the component we are interested in, and TestRenderer shows us how it behaves.
options − These are additional settings that we can offer to modify how the testing is carried out.
Return Value
After using the TestRenderer.create() it returns a TestRenderer object. This instance is what we can use to check that everything is working properly.
Examples
Example − Hello World App and its Testing
So first we will create a simple Hello World App and its corresponding testing file to use the TestRenderer.create() method and to create instances of these components and log the resulting structure to the console. So the code for this method is as follows −
HelloWorldApp.js
import React from 'react'; function HelloWorldApp() { return <div className='App'>Hello, World!</div>; } export default HelloWorldApp;
TestHelloWorldApp.js
import TestRenderer from 'react-test-renderer'; import HelloWorldApp from './HelloWorldApp'; // Create a TestRenderer instance const testInstance = TestRenderer.create(<HelloWorldApp />); // Log the result console.log(testInstance.toJSON());
Output

So we can see in the above output image there is Hello World! printed on the screen. TestHelloWorldApp file is a HelloWorldApp test file. To create an instance of the HelloWorldApp component, it utilizes the TestRenderer from the react-test-renderer package.
Example − Counter App and its Testing
Now we will create a simple React component CounterApp that shows a counter and an increment button. We also have a test file, TestCounterApp.js, that makes use of TestRenderer to create a CounterApp instance and log the resulting structure to the console.
CounterApp.js
import React, { useState } from 'react'; function CounterApp() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); } export default CounterApp;
TestCounterApp.js
import TestRenderer from 'react-test-renderer'; import CounterApp from './CounterApp'; // Create a TestRenderer instance const testInstance = TestRenderer.create(<CounterApp />); // Log the result console.log(testInstance.toJSON());
Output

TestCounterApp file is a CounterApp test file. To create an instance of the CounterApp component, it uses the TestRenderer from the react-test-renderer package. The component tree is then converted to a JSON-like structure using the toJSON() method, and the result is logged to the console.
Example − Todolist App and its Testing
Now we will create a TodoListApp. It will have a React component that provides a simple todo list. We will also have a test file, TestTodoListApp.js, which will use TestRenderer to generate a TodoListApp instance and log the resulting structure to the console. So the code for this application is mentioned below −
TodoListApp.js
import React, { useState } from 'react'; import './App.css'; function TodoListApp() { const [todos, setTodos] = useState([]); const [newTodo, setNewTodo] = useState(''); const addTodo = () => { setTodos([...todos, newTodo]); setNewTodo(''); }; return ( <div className='App'> <h2>Todo List</h2> <ul> {todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> <input type="text" value={newTodo} onChange={(e) => setNewTodo(e.target.value)} /> <button onClick={addTodo}>Add Todo</button> </div> ); } export default TodoListApp;
TestTodoListApp.js
import TestRenderer from 'react-test-renderer'; import TodoListApp from './TodoListApp'; // Create a TestRenderer instance const testInstance = TestRenderer.create(<TodoListApp />); // Log the result console.log(testInstance.toJSON());
Output

TestTodoListApp file is a TodoListApp test file. To create an instance of the TodoListApp component, it uses the TestRenderer from the react-test-renderer package.
Summary
So the TestRenderer.create() is a useful tool to create a magical mirror for our React components so that we can see how it looks without needing a real web page. And for knowing the usage of this method we have created different apps for better understanding.
To Continue Learning Please Login