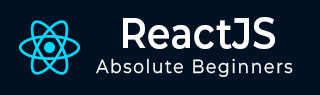
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.findByType() Method
Testing is like a hero in the programming world. It is used to check that our code behaves as expected. The findByType() function is a helpful tool in this procedure. Let us examine this function and how it helps us.
findByType() is a command that helps us find a specific test instance within a group of tests. It is like having an instructor, directing us to the accurate test we want to take.
findByType() is a useful tool for testing. This function can help us navigate a maze of tests, making sure our testing journey is both efficient and useful.
A more focused method is testInstance.findByType(type). It limits the search to a single sort of test. Define "type" to be a category or label that helps us in determining the nature of the test.
Syntax
testInstance.findByType(type)
Parameters
type − A ‘type’ defines tests based on their purpose or usage. For example, we may have tests that verify numbers, others that check strings, and so on. By specifying a type, findByType() can focus on tests that are included within the given category.
Return Value
When we call testInstance.findByType(type), the function will return a single descendent test instance that matches the type we gave.
Examples
Now we will create different React apps using testInstance.findByType(type) method for showing how this function can be used in various scenarios.
Example − Basic Component Search
Suppose we have a React app with various components, and we want to find a specific component using testInstance.findByType(type).
App.js
import React from 'react'; import ComponentA from './ComponentA'; function App() { return ( <div> <h1>React App</h1> <ComponentA /> </div> ); } export default App;
ComponentA.js
import React from 'react'; const ComponentA = () => { return ( <div> <p>This is Component A</p> </div> ); } export default ComponentA;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find ComponentA using findByType', () => { const { findByType } = render(<App />); const componentA = findByType('ComponentA'); // perform further testing expect(componentA).toBeInTheDocument(); });
Output

This example showcases a basic component search scenario in which testInstance.findByType(type) can be applied in testing React applications.
Example − Dynamic Component Rendering
In this case, let us imagine a React app in which components are dynamically rendered as per the user interactions. So the code for this app and its corresponding test case is as follows −
App.js
import React, { useState } from 'react'; import DynamicComponent from './components/DynamicComponent'; function App() { const [showComponent, setShowComponent] = useState(true); return ( <div> <h1>React App Example 2</h1> {showComponent && <DynamicComponent />} </div> ); } export default App;
DynamicComponent.js
import React from 'react'; const DynamicComponent = () => { return ( <div> <p>This is a Dynamic Component</p> </div> ); } export default DynamicComponent;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find DynamicComponent using findByType', () => { const { findByType } = render(<App />); const dynamicComponent = findByType('DynamicComponent'); // perform further testing expect(dynamicComponent).toBeInTheDocument(); });
Output

This example showcases dynamic component rendering scenario in which testInstance.findByType(type) can be applied in testing React applications.
Example − Conditional Rendering with Error Handling
In this example we will explore a React app with conditional rendering and error handling using testInstance.findByType(type). So the code for this app and its test file is as follows −
App.js
import React, { useState } from 'react'; import ErrorBoundary from './components/ErrorBoundary'; function App() { const [hasError, setHasError] = useState(false); const triggerError = () => { setHasError(true); }; return ( <div> <h1>React App Example 3</h1> <ErrorBoundary hasError={hasError} /> <button onClick={triggerError}>Trigger Error</button> </div> ); } export default App;
ErrorBoundary.js
import React from 'react'; class ErrorBoundary extends React.Component { componentDidCatch(error, info) { // Handle the error console.error('Error caught:', error, info); } render() { if (this.props.hasError) { // Render fallback UI in case of an error return <p>Something went wrong!</p>; } return this.props.children; } } export default ErrorBoundary;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find ErrorBoundary using findByType', () => { const { findByType } = render(<App />); const errorBoundary = findByType('ErrorBoundary'); // perform further testing expect(errorBoundary).toBeInTheDocument(); });
Output

This example showcases Conditional Rendering with Error Handling scenario in which testInstance.findByType(type) can be applied in testing React applications.
Summary
testInstance.findByType(type) limits the search to a specific type of test. The result of this method returns the specific test instance of the provided type, assuming a unique match. If there are any errors, it will notify us with an error message, prompting us to look into and modify our request or verify the organization of our tests.
Remember that the function tries for accuracy, and its return is conditional on getting a clear, unique match for the given type.
To Continue Learning Please Login