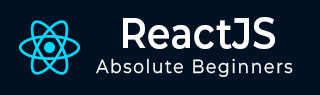
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - testInstance.findAllByType() Method
In software testing, we often need to locate specific test instances based on their type. The testInstance.findAllByType() method can help us with this. This function is mainly used to find all test instances without specifying a particular type.
It searches for and returns all descendant test instances, regardless of their type. If we have a specific type in mind, we can use this function. It helps to find and return all test instances with the specified type.
These methods make it easier for software developers and testers to locate and work with test instances based on their types.
Syntax
testInstance.findAllByType(type)
Parameters
type − This is the parameter we provide when calling the findAllByType method. It shows the specific type of test instances we want to find. We need to replace the type with the actual type we are looking for.
Return Value
This method returns a collection of test instances that match the specified type. The collection contains all descendant test instances with the given type.
Examples
So now we will create different apps and its test cases to better understand the testInstance.findAllByType() method.
Example − Basic Component Search
Let us say we have a simple Button component that we want to test. Now, we can use the Button component in our main App component. Make sure to adjust the path to the Button component based on the project structure. This example show how to test the Button component using findAllByType in a simple manner.
Button.js
import React from 'react'; const Button = ({ label }) => { return ( <button>{label}</button> ); }; export default Button;
App.js
import React from 'react'; import { create } from 'react-test-renderer'; import Button from './Button'; const App = () => { // Create a test renderer instance for the component const testRenderer = create(<Button label="Click me" />); // Get the root test instance const testInstance = testRenderer.root; // Now we can use findAllByType const foundInstances = testInstance.findAllByType('button'); return ( <div> <h1>Basic Component Search</h1> <p>Found {foundInstances.length} instances of Button component.</p> </div> ); }; export default App;
Output

As we can see in the above output image. There is a message visible and in that it is written that we have found a button component in the app. This is a basic component search operation where we can use findAllByType() method.
Example − Custom Component Search
Let us say we have a simple custom component named CustomComponent. Now we can use this CustomComponent in our App component. Make sure to adjust the path to the CustomComponent component based on the project structure. This example shows how to test the CustomComponent using findAllByType in a simple way.
CustomComponent.js
import React from 'react'; const CustomComponent = ({ text }) => { return ( <div> <p>{text}</p> </div> ); }; export default CustomComponent;
App.js
import React from 'react'; import { create } from 'react-test-renderer'; import CustomComponent from './CustomComponent'; const App = () => { // Create a test renderer instance for the component const testRenderer = create(<CustomComponent text="Hello, Custom Component!" />); // Get the root test instance const testInstance = testRenderer.root; // Now we can use findAllByType const customComponentType = CustomComponent; const foundInstances = testInstance.findAllByType((node) => node.type === customComponentType); return ( <div> <h1>Custom Component Search</h1> <p>Found {foundInstances.length} instances of CustomComponent component.</p> </div> ); }; export default App;
Output

The actual output will vary depending on the testing configuration, and this is only an example. The primary goal of these tests is to make sure that the components behave as supposed and that the test assertions are valid.
Example − Dynamic Component Search
Below is an example of another React app using testInstance.findAllByType(type). This app renders a list of items and allows us to search for a particular item type dynamically. The ItemList component renders a list of items, and the App component dynamically changes the item type and logs the number of instances found as per the selected item type.
ItemList.js
import React from 'react'; const ItemList = ({ items }) => { return ( <div> <h2>Item List</h2> <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> </div> ); }; export default ItemList;
App.js
import React, { useState, useEffect } from 'react'; import { create } from 'react-test-renderer'; import ItemList from './ItemList'; const App = () => { const [itemType, setItemType] = useState('Fruit'); useEffect(() => { // Creating a test renderer instance const testRenderer = create(<ItemList items={['Apple', 'Banana', 'Orange']} />); const testInstance = testRenderer.root; // Finding instances dynamically const foundInstances = testInstance.findAllByType('li'); console.log(`Found ${foundInstances.length} instances of ${itemType} in the list.`); }, [itemType]); return ( <div> <h1>Dynamic Item Search</h1> <p>Change item type dynamically:</p> <button onClick={() => setItemType('Fruit')}>Show Fruits</button> <button onClick={() => setItemType('Vegetable')}>Show Vegetables</button> </div> ); }; export default App;
Output

Summary
So overall we can say that when we use findAllByType(type), we need to tell it what type of test instances we are interested in (the type parameter), and in return, it gives us a group of those specific test instances. And we have created three different apps based on different situations like basic component search, custom component search and dynamic component search. You can use this function as per your specific requirements.
To Continue Learning Please Login