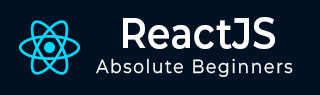
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - findRenderedDOMComponentWithTag()
In React, there is a useful function called findRenderedDOMComponentWithTag(). This function works like a special detective, searching through our app's virtual world for the particular component known as a tag.
When we use findRenderedDOMComponentWithTag(), we are telling our computer program to locate a specific tag in the application's virtual representation, which is known as the DOM (Document Object Model).
The function checks for that exact tag. The most interesting part is that it expects to find exactly one match. If there are more than one or none, it throws an exception.
Syntax
findRenderedDOMComponentWithTag( tree, tagName )
Parameters
tree − This acts as a map of our virtual environment, displaying all of the elements and how they are linked.
tagName − It is the name of the tag we are looking for in our virtual environment.
Return Value
The function findRenderedDOMComponentWithTag() gives a single result. When we use this function, we are telling our computer program to locate a specific HTML tag (like div>, <span>, etc.) in our app's DOM. The method expects to identify only one match for the provided tag. If it detects more than one or none, it throws an exception, indicating that something is not as it should be.
Examples
Example − Find a Heading
Let us say we have a virtual book, and we want to find the title, which is a heading (h1) tag. So for doing this task we will use findRenderedDOMComponentWithTag(tree, 'h1'). So below is the code for this application −
// Import required libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <h1>Title of the Book</h1> </div> ); }; // Test case const { container } = render(<App1 />); const headingElement = findRenderedDOMComponentWithTag(container, 'h1'); console.log(headingElement.textContent);
Output
Title of the Book
The goal of this program is to find and display that title by using the findRenderedDOMComponentWithTag() function. When we ask the application to locate the heading (h1 tag), it should return the phrase "Title of the Book."
Example − Locating Buttons
Let's imagine we have a bunch of buttons in this application. To find and interact with a specific button, use findRenderedDOMComponentWithTag(tree, 'button').
// Import necessary libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <button onClick={() => console.log('Button 1 is clicked')}>Button 1</button> <button onClick={() => console.log('Button 2 is clicked')}>Button 2</button> </div> ); }; // Test case const { container } = render(<App />); const buttonElement = findRenderedDOMComponentWithTag(container, 'button'); buttonElement.click();
Output
Button 1 is clicked
There are two buttons in the program, one labeled "Button 1" and the other "Button 2." The goal here is to find and interact with these buttons using the findRenderedDOMComponentWithTag() function. When we press the button, it should display a message such as "Button 1 is clicked."
Example 3
Let's create an application in which we have some images. To locate a specific image we will use findRenderedDOMComponentWithTag(tree, 'img').
// Import required libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <img src="image1.jpg" alt="This is Image 1" /> <img src="image2.jpg" alt="This is Image 2" /> </div> ); }; // Test case const { container } = render(<App />); const imageElement = findRenderedDOMComponentWithTag(container, 'img'); console.log(imageElement.getAttribute('alt'));
Output
This is Image 1
In this application there are two photos, each of which shows something different. The aim is to identify and display information about these images using the findRenderedDOMComponentWithTag() function. When we find an image, for example, it should inform us what it is. If it is the first image, it can say something like, "This is Image 1."
Summary
The findRenderedDOMComponentWithTag() method acts as a digital explorer, helping us in locating specific items in our app's virtual environment. It ensures we get exactly what we want. So this feature makes our coding experience easy. Remember to use it carefully and expect it to find only one thing. It will notify us if there is more or less.
To Continue Learning Please Login