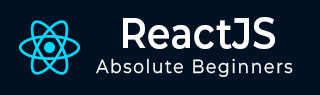
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - findRenderedDOMComponentWithClass()
In ReactJS, the findRenderedDOMComponentWithClass() method is used for testing. It helps us in finding a rendered component in the DOM with a particular CSS class. This method is mainly used to test React components.
When writing tests for our React components, we can check to see if a component with a given CSS class appears in the rendered output. That is exactly what findRenderedDOMComponentWithClass() does. So we pass this function the rendered component and the CSS class we want. The first DOM element with that class is then returned.
Remember that this function was more commonly used in previous versions of React and testing frameworks. More current testing libraries can offer different methods to get the same goal.
Syntax
findRenderedDOMComponentWithClass( tree, className )
Parameters
tree − It is the displayed component or component tree inside which we want to search. It is the rendered output of our React component, as received from a testing library.
className − A string that represents the CSS class we want to find in the tree. The method will search for a DOM element with this particular class.
Return Value
The method will return the first DOM element with the given class, allowing us to continue our tests with additional actions or assertions.
Examples
Example − Basic React App
App Explanation: This is like a web page showing “Hello, React!” on the webpage. Imagine it is a simple greeting card.
Testing Explanation − We want to make sure a specific part of the greeting card (the paragraph) is there. So, we use a tool to find that part and check if it is what we want.
App.js
import React from 'react'; import './App.css'; function App() { return ( <div className="app-container App"> <h1>Hello, React!</h1> <p className="app-paragraph">This is a simple React app.</p> </div> ); } export default App;
App.test.js
import { render } from '@testing-library/react'; import findRenderedDOMComponentWithClass from 'path-to-findRenderedDOMComponentWithClass'; import App from './App'; test('finds a paragraph with a specific class', () => { const { container } = render(<App />); const foundElement = findRenderedDOMComponentWithClass(container, 'app-paragraph'); // Now we can make assertions or tests based on foundElement });
Output

Example − React App with Components
App Explanation − In the app suppose we have a webpage and the webpage has a title (header) and a paragraph. It is like a small website with a title at the top.
Testing Explanation − We want to make sure the title is there. So, we use a tool to find the title and check if it is in the right place.
Header.js
import React from 'react'; function Header() { return <h1 className="header-title">Welcome to My App</h1>; } export default Header;
App.js
import React from 'react'; import Header from './Header'; import './App.css'; export default function App() { return ( <div className="app-container"> <Header /> <p className="app-paragraph">This app has a header component.</p> </div> ); }
App.test.js
import { render } from '@testing-library/react'; import findRenderedDOMComponentWithClass from 'path-to-findRenderedDOMComponentWithClass'; import App from './App'; test('finds a header with a specific class', () => { const { container } = render(<App />); const foundElement = findRenderedDOMComponentWithClass(container, 'header-title'); });
Output

Example − React App with Dynamic Content
App Explanation − This is like a webpage that can show different messages. It is a bit like a signboard that can display changing information.
Testing Explanation − We want to make sure the changing message is there. So, we use a tool to find that part and check if it is showing the correct message.
DynamicContent.js
import React from 'react'; function DynamicContent({ content }) { return <div className="dynamic-content">{content}</div>; } export default DynamicContent;
App.js
import React from 'react'; import DynamicContent from './DynamicContent'; import './App.css'; function App() { return ( <div className="app-container App"> <DynamicContent content="This content is dynamic!" /> <p className="app-paragraph">This app includes dynamic content.</p> </div> ); }
App.test.js
import { render } from '@testing-library/react'; import findRenderedDOMComponentWithClass from 'path-to-findRenderedDOMComponentWithClass'; import App from './App'; test('finds a dynamic content with a specific class', () => { const { container } = render(<App />); const foundElement = findRenderedDOMComponentWithClass(container, 'dynamic-content'); });
Output

Summary
This function is used in testing for React components. It helps us find a specific element in the finished result of our React component when we are running tests. Suppose we have a photo of a group of people and we want to find someone wearing a red hat, we will use this function to locate that person in the picture. It is a useful tool for testing to make sure our components are behaving as expected.
To Continue Learning Please Login