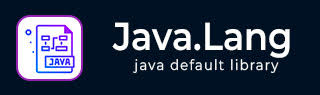
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Long compareTo() method
Description
The Java Long compareTo() method compares two Long objects numerically.
Declaration
Following is the declaration for java.lang.Long.compareTo() method
public int compareTo(Long anotherLong)
Parameters
anotherLong − This is the Long to be compared.
Return Value
This method returns the value 0 if this Long is equal to the argument Long, a value less than 0 if this Long is numerically less than the argument Long and a value greater than 0 if this Long is numerically greater than the argument Long.
Exception
NA
Comparing Two Long Objects with Positive Values Example
The following example shows the usage of Long compareTo() method to compare two Long objects. We've created two Long variables and assigned them Long objects created using a positive long values. Then using compareTo() method, we're comparing the Long objects.
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { // compares two Long objects numerically Long obj1 = new Long("25"); Long obj2 = new Long("10"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is greater than obj2
Comparing Two Long Objects with Negative Values Example
The following example shows the usage of Long compareTo() method to compare two Long objects. We've created two Long variables and assigned them Long objects created using a negative long values. Then using compareTo() method, we're comparing the Long objects.
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { // compares two Long objects numerically Long obj1 = new Long("-25"); Long obj2 = new Long("-10"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is less than obj2
Comparing Two Long Objects with Same Values Example
The following example shows the usage of Long compareTo() method to compare two Long objects. We've created two Long variables and assigned them Long objects created using a same positive long values. Then using compareTo() method, we're comparing the Long objects.
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { // compares two Long objects numerically Long obj1 = new Long("25"); Long obj2 = new Long("25"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is equal to obj2
To Continue Learning Please Login